java实验学生选课系统
时间: 2023-09-02 12:14:16 浏览: 90
选课系统的实现可以分为以下几个步骤:
1. 定义学生和课程类
```java
public class Student {
private String name;
private int id;
private List<Course> courses;
// 构造函数、getter和setter方法
}
public class Course {
private String name;
private int id;
private int credit;
private int capacity;
private List<Student> students;
// 构造函数、getter和setter方法
}
```
2. 实现选课和退课功能
```java
public class CourseSelectionSystem {
private List<Student> students;
private List<Course> courses;
public void selectCourse(Student student, Course course) {
if (course.getCapacity() > 0) {
course.getStudents().add(student);
student.getCourses().add(course);
course.setCapacity(course.getCapacity() - 1);
} else {
System.out.println("选课失败,课程已满!");
}
}
public void dropCourse(Student student, Course course) {
course.getStudents().remove(student);
student.getCourses().remove(course);
course.setCapacity(course.getCapacity() + 1);
}
// 其他方法
}
```
3. 编写测试类
```java
public class TestCourseSelectionSystem {
public static void main(String[] args) {
Course math = new Course("高等数学", 1, 3, 2);
Course english = new Course("英语", 2, 2, 1);
Student alice = new Student("Alice", 1);
Student bob = new Student("Bob", 2);
CourseSelectionSystem system = new CourseSelectionSystem();
system.selectCourse(alice, math);
system.selectCourse(bob, math);
system.selectCourse(bob, english);
system.dropCourse(alice, math);
}
}
```
以上是一个简单的选课系统的实现,可以根据需求进行扩展。
阅读全文
相关推荐

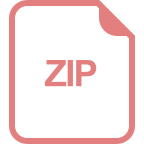
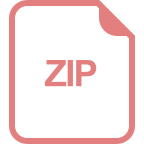
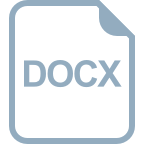
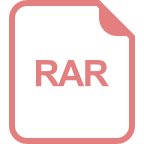
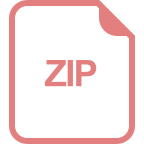
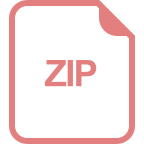
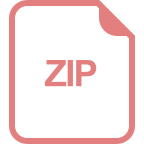
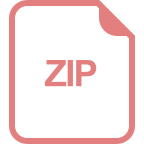
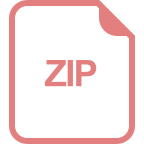
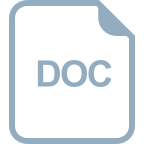
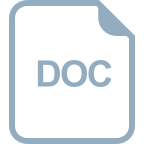
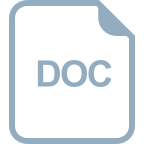
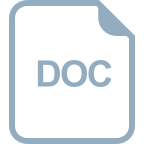
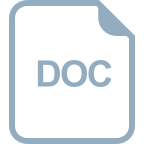