react hooks 将数据存入redux
时间: 2023-07-21 17:03:34 浏览: 136
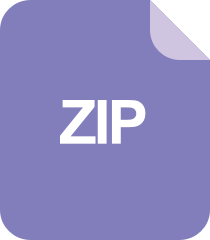
hooks:在react-hooks中使用redux
React Hooks 是一种 React 新特性,可以让函数组件具有类组件的功能,包括状态管理。而 Redux 则是一种全局状态管理工具,用于管理整个应用的状态。
要将数据存入 Redux,你需要使用 Redux 的 API,包括 createStore、combineReducers、dispatch 等来创建和管理 store,然后使用 useSelector 和 useDispatch Hooks 来获取和更新 store 中的数据。
下面是一个示例:
1. 创建 Redux store
```javascript
import { createStore } from 'redux';
// 定义 reducer
const counterReducer = (state = { count: 0 }, action) => {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
};
// 创建 store
const store = createStore(counterReducer);
```
2. 在组件中使用 useSelector 和 useDispatch Hooks
```javascript
import { useSelector, useDispatch } from 'react-redux';
const Counter = () => {
const count = useSelector(state => state.count);
const dispatch = useDispatch();
const handleIncrement = () => {
dispatch({ type: 'INCREMENT' });
};
const handleDecrement = () => {
dispatch({ type: 'DECREMENT' });
};
return (
<div>
<h1>Count: {count}</h1>
<button onClick={handleIncrement}>+</button>
<button onClick={handleDecrement}>-</button>
</div>
);
};
```
在上面的代码中,我们使用 useSelector Hook 获取 store 中的 count 数据,并使用 useDispatch Hook 获取 dispatch 函数,以便更新 store 中的数据。
当点击按钮时,我们分别调用 handleIncrement 和 handleDecrement 函数来分发 INCREMENT 和 DECREMENT action,从而更新 store 中的 count 数据。
阅读全文
相关推荐
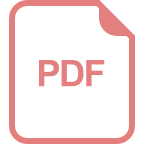
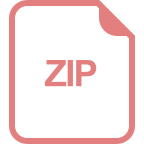
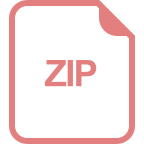
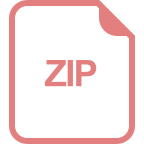
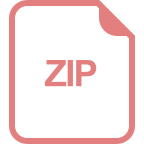
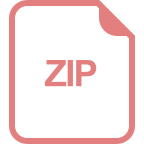
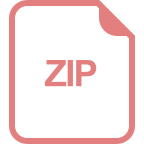
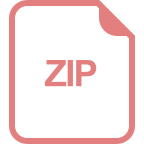
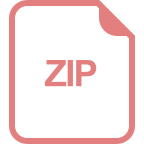
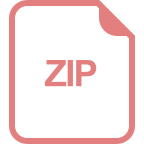
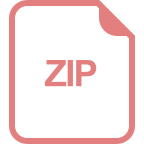
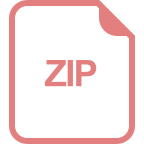
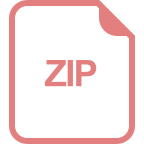
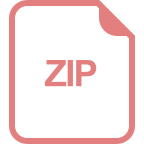
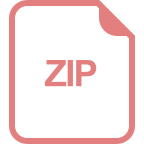
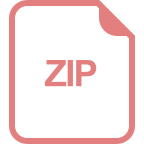
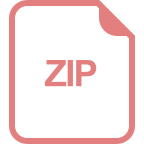