用c++实现用线性探测法解决散列表冲突源码加注释
时间: 2024-01-04 13:04:33 浏览: 97
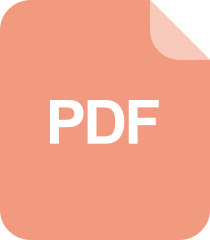
数据结构实验C语言实现散列表-构造-解决冲突-查找.pdf
好的,下面是使用线性探测法解决散列表冲突的 C++ 代码,已经加上注释:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
const int TABLE_SIZE = 10; // 散列表的大小
const int MAX_NUM = 20; // 随机生成的数字的最大值
class HashEntry {
private:
int key; // 键值
int value; // 实际存储的值
public:
HashEntry(int key, int value) {
this->key = key;
this->value = value;
}
int getKey() {
return key;
}
int getValue() {
return value;
}
};
class HashMap {
private:
HashEntry **table; // 散列表
public:
HashMap() {
table = new HashEntry*[TABLE_SIZE];
for (int i = 0; i < TABLE_SIZE; i++)
table[i] = NULL;
}
// 计算散列值
int hashFunc(int key) {
return key % TABLE_SIZE;
}
// 插入元素
void insert(int key, int value) {
int hash = hashFunc(key);
while (table[hash] != NULL && table[hash]->getKey() != key) {
hash = (hash + 1) % TABLE_SIZE;
}
if (table[hash] != NULL) {
delete table[hash];
}
table[hash] = new HashEntry(key, value);
}
// 查找元素
int get(int key) {
int hash = hashFunc(key);
while (table[hash] != NULL && table[hash]->getKey() != key) {
hash = (hash + 1) % TABLE_SIZE;
}
if (table[hash] == NULL) {
return -1;
} else {
return table[hash]->getValue();
}
}
// 删除元素
void remove(int key) {
int hash = hashFunc(key);
while (table[hash] != NULL) {
if (table[hash]->getKey() == key)
break;
hash = (hash + 1) % TABLE_SIZE;
}
if (table[hash] == NULL) {
cout << "没有找到要删除的元素!" << endl;
return;
} else {
delete table[hash];
table[hash] = NULL;
}
}
~HashMap() {
for (int i = 0; i < TABLE_SIZE; i++) {
if (table[i] != NULL) {
delete table[i];
}
}
delete[] table;
}
};
int main() {
// 创建散列表
HashMap hash;
// 插入随机数
srand(time(NULL));
for (int i = 0; i < TABLE_SIZE; i++) {
int key = rand() % MAX_NUM;
int value = rand() % MAX_NUM;
cout << "插入元素:key = " << key << " value = " << value << endl;
hash.insert(key, value);
}
// 查找元素
for (int i = 0; i < MAX_NUM; i++) {
int value = hash.get(i);
if (value == -1)
cout << "没有找到元素:" << i << endl;
else
cout << "找到元素:key = " << i << " value = " << value << endl;
}
// 删除元素
for (int i = 0; i < MAX_NUM; i++) {
cout << "删除元素:" << i << endl;
hash.remove(i);
}
return 0;
}
```
注释已经在代码中,希望能对你有所帮助!
阅读全文
相关推荐
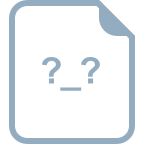
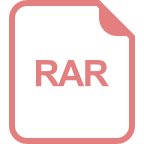
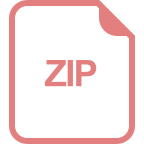
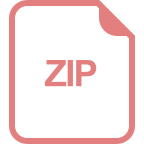
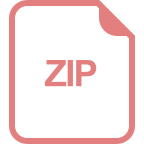
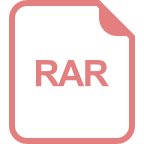
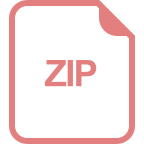
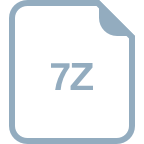
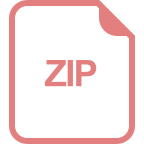
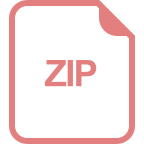
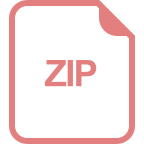
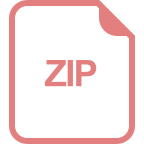
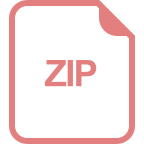
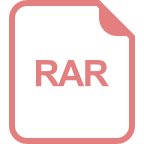