systemverilog中extends
时间: 2023-09-15 09:20:07 浏览: 77
在SystemVerilog中,`extends`关键字用于创建一个继承类(子类)。子类可以继承父类中的属性和方法,并且可以添加自己的属性和方法。
下面是一个简单的示例:
```
class Animal;
int age;
function void makeSound();
$display("The animal makes a sound");
endfunction
endclass
class Dog extends Animal;
function void makeSound();
$display("The dog barks");
endfunction
endclass
module test;
Dog myDog = new();
initial begin
myDog.age = 3;
myDog.makeSound();
end
endmodule
```
在上面的示例中,`Dog`是一个继承自`Animal`的子类。它继承了`Animal`类中的`age`属性和`makeSound()`方法,并且添加了自己的`makeSound()`方法。在`test`模块中,我们创建了一个`Dog`对象并调用了它的`makeSound()`方法。
输出结果为:
```
The dog barks
```
相关问题
systemverilog中的oop
SystemVerilog支持基于对象的编程(OOP)范例。SystemVerilog中的对象是一个包含数据和方法的实体。对象是基于一个类的定义创建的,类定义了对象可以拥有的属性和行为。
SystemVerilog中的OOP主要包括以下几个方面:
1. 类定义:使用class关键字来定义一个类,可以定义类的属性和方法。
2. 对象创建:使用new方法来创建一个对象,可以使用对象的构造函数来初始化对象。
3. 继承:使用extends关键字来实现继承,子类可以继承父类的属性和方法。
4. 多态:使用virtual和override关键字来实现多态,可以让子类重写父类的方法。
5. 访问控制:使用public、private和protected关键字来控制类的属性和方法的访问权限。
通过OOP可以实现面向对象的设计,可以提高代码的可重用性和可维护性。在SystemVerilog中,OOP的使用也可以提高代码的可读性和可扩展性。
systemverilog中类的多态
SystemVerilog中的多态(polymorphism)特性是指子类和父类之间多个子程序使用同一个名字的现象。当子类从父类扩展创建之后,子类就继承了父类的属性和方法,这是SystemVerilog的继承特性。然而,要使用多态特性,需要遵循以下规则:
1. 子类的方法必须与父类的方法具有相同的名称和参数列表。
2. 子类的方法可以重写(override)父类的方法,即在子类中重新定义方法的实现。
3. 子类的方法可以通过super关键字调用父类的方法。
4. 子类的方法可以通过this关键字调用自身的其他方法。
通过使用多态特性,可以在不同的子类对象上调用相同的方法,但实际执行的是各自子类中的方法实现。这样可以提高代码的灵活性和可扩展性。
以下是一个示例代码,演示了SystemVerilog中类的多态特性:
```systemverilog
class Animal;
virtual function void makeSound();
$display("Animal makes sound");
endfunction
endclass
class Dog extends Animal;
function void makeSound();
$display("Dog barks");
endfunction
endclass
class Cat extends Animal;
function void makeSound();
$display("Cat meows");
endfunction
endclass
module Test;
initial begin
Animal a;
Dog d;
Cat c;
a = new;
d = new;
c = new;
a.makeSound(); // 输出:Animal makes sound
d.makeSound(); // 输出:Dog barks
c.makeSound(); // 输出:Cat meows
end
endmodule
```
在上述示例中,Animal类是父类,Dog和Cat类是子类。它们都有一个名为makeSound的方法。通过创建不同的子类对象,并调用它们的makeSound方法,可以看到每个子类对象都执行了自己的方法实现,实现了多态特性。
相关推荐
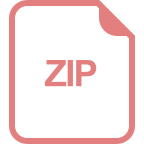












