import java.io.*; import javax.servlet.*; import javax.servlet.http.*, public class HelloCounter extends HttpServlet{ private int visits=0: public void doGet (HttpServletRequest request, HttpServletResponse response) throws ServletException,IOExceptions{ response.setContentType ("text/html; charset=\"UTF-8\""); PrintWriter servletOut = response.getWriter(); visits++; PrintVisitNum(servletOut, new Integer(visits).toString()); servletOut.close(); } private void PrintVisitNum(PrintWriter servletOut,String visits){ //we assume that the codes have been rightly implemented to print the visits and they are omitted here.} }Modify the HelloCounter servlet and rename it the VisitorCounter servlet. In the VisitorCounter servlet, you are required to create a session so that the number of visitors to the page, rather than the number of visits, is displayed. Note that you are only required to explain how the code should be modified and write the modified code accordingly.
时间: 2023-11-28 12:51:28 浏览: 104
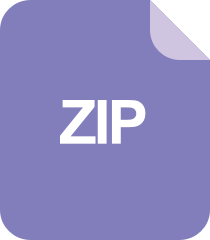
Java实现简单文件下载共3页.pdf.zip
To modify the HelloCounter servlet into the VisitorCounter servlet with session tracking, you can make the following changes:
1. Rename the class from HelloCounter to VisitorCounter.
2. In the doGet method, retrieve the session object from the request using request.getSession() method.
3. Within the session object, retrieve the visitor count attribute using session.getAttribute("count"), and if it does not exist, set it to 0 using session.setAttribute("count", 0).
4. Increment the visitor count using session.setAttribute("count", count + 1).
5. Print the visitor count to the response using the PrintWriter object.
6. Close the PrintWriter object.
The modified code would look like this:
```
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class VisitorCounter extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html; charset=\"UTF-8\"");
PrintWriter servletOut = response.getWriter();
HttpSession session = request.getSession();
Integer count = (Integer) session.getAttribute("count");
if (count == null) {
count = 0;
}
count += 1;
session.setAttribute("count", count);
servletOut.println("<html><head><title>Visitor Counter</title></head><body>");
servletOut.println("<h1>Visitor Count: " + count + "</h1>");
servletOut.println("</body></html>");
servletOut.close();
}
}
```
Note: This code assumes that the web.xml file has been correctly configured to map the servlet to a URL.
阅读全文
相关推荐
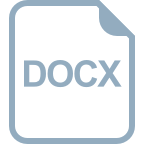
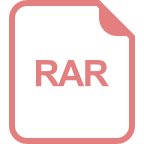















