How to use pytest to test a complete pyqt5 App
时间: 2024-11-13 13:27:50 浏览: 22
To use `pytest` to test a complete PyQt5 application, you'll first need to install the `pytest-qt` plugin, as it provides essential tools for testing Qt applications. You can install it using pip:
```shell
pip install pytest-qt
```
Here's an overview of how to structure your testing with pytest and pytest-qt:
1. **Set up fixtures**: Use pytest fixtures to initialize your Qt application before each test case. This could involve creating an instance of your main window or any required components[^1].
```python
import pytest
from your_app_window import YourAppWindow
@pytest.fixture
def app_window(qtbot):
app = QApplication([])
window = YourAppWindow()
qtbot.addWidget(window)
yield window
window.close()
app.quit()
```
2. **Test event handling**: pytest-qt allows controlling the Qt event loop during tests. For example, if your application relies on signals, you may want to call `app.processEvents()` to let events be processed.
```python
def test_signal_handling(app_window, qtbot):
button = app_window.button
button.click()
qtbot.waitUntil(lambda: app_window.signal_emitted, timeout=1000) # Wait for signal to be emitted
```
3. **Integration tests**: Write end-to-end tests that simulate user interactions and verify expected results.
```python
def test_menu_functionality(app_window, qtbot):
menu_item = app_window.menu_bar.actions()[0]
qtbot.mouseClick(menu_item, QtCore.Qt.LeftButton)
assert app_window.some_result == expected_value
```
4. **Requirements management**: Keep your `requirements.txt` file updated with all necessary dependencies, including `PySide6`[^2].
Remember to include relevant imports in your test modules, like `from pytest_qt import qtbot`, and ensure that your tests are decorated with `@pytest.mark.parametrize` or other appropriate mechanisms to cover different scenarios.
阅读全文
相关推荐












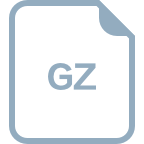



