利用数据结构教程设计一个算法,查找带头节点的非空单链表l中的最小结点(最小节点可能有多个),并返回该节点的逻辑序号
时间: 2024-11-09 17:23:18 浏览: 65
为了在一个带头节点的非空单链表`l`中查找最小元素及其逻辑顺序,我们可以采用迭代的方式遍历整个链表。以下是设计的一个简单算法:
1. **初始化**:假设头节点为`head`,初始最小值为`head.data`,逻辑顺序为0,当前指针为`current = head`。
2. **遍历链表**:遍历时,将当前节点的数据与已知最小值比较。如果当前节点的数据更小,更新最小值和逻辑顺序。
```python
while current is not None:
if current.data < min_value:
min_value = current.data
min_index = logic_order
logic_order += 1
current = current.next
```
3. **返回结果**:遍历结束后,`min_value`即为最小元素,`min_index`为其逻辑顺序。
**伪代码如下:**
```plaintext
function findSmallestNode(head):
min_value = head.data
min_index = 0
current = head.next
while current is not None:
if current.data < min_value:
min_value = current.data
min_index = current.prev.next.index(current) + 1 # 获取逻辑顺序(考虑到头节点)
current = current.next
return (min_value, min_index)
```
在这个算法中,我们通过计算当前节点在其前驱节点之后的位置(加1是因为我们要排除头节点)来得到逻辑顺序。
阅读全文
相关推荐
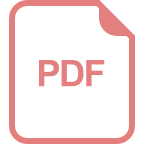
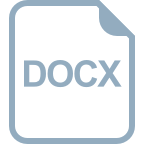
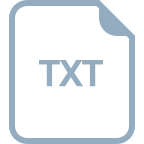
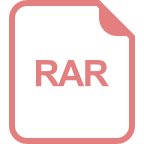
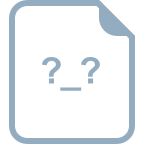
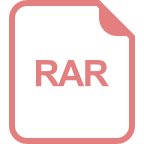
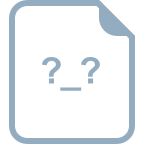
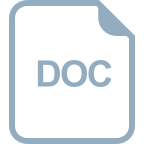
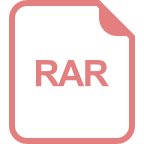
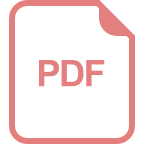
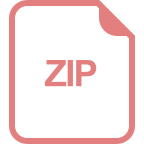
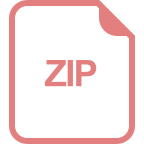
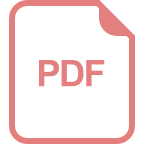
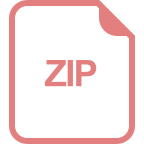
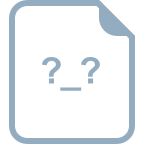
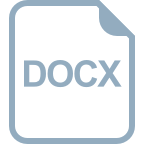