Python如何给图片去重
时间: 2024-11-11 13:30:15 浏览: 23
在Python中,有多种方法可以检测并去除图片的重复内容,特别是在处理大量图像数据时,这通常是通过比较图片的特征来进行的。以下是几种常见的技术:
1. **基于像素值**:
使用`PIL` (Pillow) 库读取图片,然后将像素数据转换成哈希值或者直方图。如果两个图片的像素值相似度足够高,就认为它们是重复的。
```python
from PIL import Image
import hashlib
def image_hash(image_path):
with Image.open(image_path) as img:
data = img.getdata()
return hashlib.md5(data).hexdigest()
# 检查图片是否重复
images = ['image1.jpg', 'image2.jpg']
unique_images = set()
for img in images:
if image_hash(img) not in unique_images:
unique_images.add(image_hash(img))
```
2. **基于深度学习**:
更专业的做法是使用深度学习模型,如卷积神经网络(CNN),来提取图片的特征向量。像`TensorFlow`或`PyTorch`这样的库提供工具来训练这类模型。然后计算特征向量的余弦相似度来判断图片是否重复。
```python
import tensorflow as tf
from tensorflow_hub import load
model_url = "https://tfhub.dev/google/tf2-preview/image-feature-vector/4"
feature_extractor = load(model_url)
def extract_features(image_path):
feature_vector = feature_extractor(image_path)
return feature_vector.numpy()
features = [extract_features(img_path) for img_path in images]
duplicates = [i for i, feat in enumerate(features) if feat in features[:i]]
```
阅读全文
相关推荐
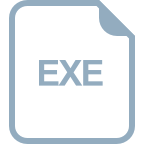
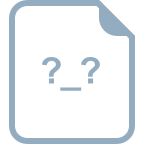
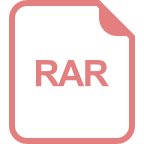
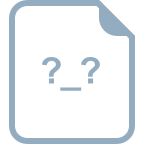

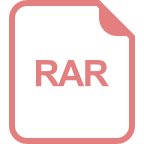
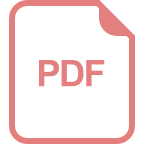
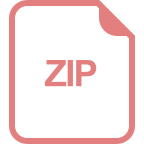
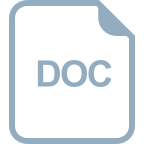
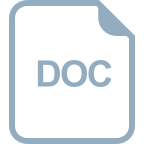



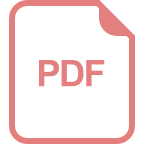
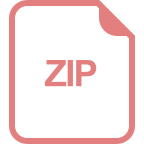
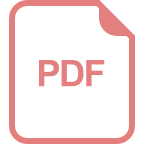

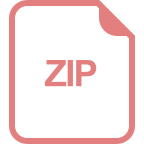
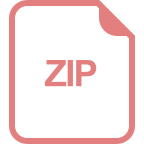