image = cv2.imread(args["image"])
时间: 2024-09-11 11:08:34 浏览: 99
这句话是在使用OpenCV(cv2库)读取图像文件,其中`args["image"]`通常是从命令行解析器(如argparse或sys.argv)中获取的一个参数。`imread`函数是OpenCV中的一个核心功能,用于从指定路径读取图像。这里的`image`变量会保存图片的内容,如果文件存在并且可以成功读取,那么`image`就是一个二维的numpy数组,表示图片的像素数据。
例如,如果你有一个命令行脚本,用户可以通过`--image`选项提供一个图片文件,那么`args["image"]`就是这个选项的值,程序会用它去调用`cv2.imread`函数读取图片。
```python
import cv2
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('--image', required=True, type=str, help='path to the image file')
args = parser.parse_args()
image_path = args.image
image = cv2.imread(image_path)
# 然后你可以对image做进一步的操作...
```
相关问题
from skimage.segmentation import slic from skimage.segmentation import mark_boundaries from skimage.util import img_as_float import matplotlib.pyplot as plt import numpy as np import cv2 import os # args args = {"image": 'I:\\18Breakageratecalculation\\SVM run\\images\\030.jpg'} # load the image and apply SLIC and extract (approximately) # the supplied number of segments image = cv2.imread(args["image"]) segments = slic(img_as_float(image), n_segments=100, sigma=3) # show the output of SLIC fig = plt.figure('Superpixels') ax = fig.add_subplot(1, 1, 1) ax.imshow(mark_boundaries(img_as_float(cv2.cvtColor(image, cv2.COLOR_BGR2RGB)), segments)) plt.axis("off") plt.show() print("segments:\n", segments) print("np.unique(segments):", np.unique(segments)) # loop over the unique segment values for (i, segVal) in enumerate(np.unique(segments)): # construct a mask for the segment print("[x] inspecting segment {}, for {}".format(i, segVal)) mask = np.zeros(image.shape[:2], dtype="uint8") mask[segments == segVal] = 255 # apply the mask to the image masked_image = np.multiply(image, cv2.cvtColor(mask, cv2.COLOR_GRAY2BGR) > 0) # save the masked image as a file filename = os.path.join(r"I:\18Breakageratecalculation\SVM run\run-images2\030", "segment_%d.png" % i) cv2.imwrite(filename, masked_image) # show the masked region cv2.imshow("Mask", mask) cv2.imshow("Applied", np.multiply(image, cv2.cvtColor(mask, cv2.COLOR_GRAY2BGR) > 0)) cv2.waitKey(0)保存超像素识别结果图像
可以使用下面的代码来保存超像素识别结果图像:
```
# create a directory to store the segmented images
if not os.path.exists("segmented_images"):
os.makedirs("segmented_images")
# save the image with superpixel boundaries
plt.imsave(os.path.join("segmented_images", "superpixel_boundaries.png"), mark_boundaries(img_as_float(cv2.cvtColor(image, cv2.COLOR_BGR2RGB)), segments))
# loop over the unique segment values
for (i, segVal) in enumerate(np.unique(segments)):
# construct a mask for the segment
mask = np.zeros(image.shape[:2], dtype="uint8")
mask[segments == segVal] = 255
# apply the mask to the image
masked_image = np.multiply(image, cv2.cvtColor(mask, cv2.COLOR_GRAY2BGR) > 0)
# save the masked image as a file
filename = os.path.join("segmented_images", "segment_%d.png" % i)
cv2.imwrite(filename, masked_image)
```
这将在当前目录下创建一个名为 "segmented_images" 的文件夹,并将超像素边界图像和每个超像素块保存为单独的图像文件。
import cv2 import torch import matplotlib.pyplot as plt from skimage.segmentation import slic from matplotlib.patches import Rectangle # 定义超像素数目 n_segments = 25 # 加载输入图像 args = {"image": r"D:\Users\Administrator\PycharmProjects\pythonProject\heart_dataset\1_blur\img-00003-00007.jpg"} # load the image and apply SLIC and extract (approximately) # the supplied number of segments image = cv2.imread(args["image"]) # 使用SLIC算法进行超像素分割 segments = slic(image.numpy().transpose((1, 2, 0)), n_segments=n_segments) # 将超像素图像转换为掩膜 mask = torch.zeros_like(image[0, :, :]) for i in range(n_segments): mask[segments == i] = i + 1 # 对掩膜进行处理,得到每个超像素块的区域 regions = [] for i in range(1, n_segments + 1): region = (mask == i).nonzero() if region.size(0) > 0: regions.append(region) # 绘制超像素块的区域 fig, ax = plt.subplots(1) ax.imshow(img.numpy().transpose((1, 2, 0))) for region in regions: x_min, y_min = region.min(dim=0)[0] x_max, y_max = region.max(dim=0)[0] rect = Rectangle((y_min, x_min), y_max - y_min, x_max - x_min, linewidth=1, edgecolor='r', facecolor='none') ax.add_patch(rect) plt.show()上述代码出现问题: segments = slic(image.numpy().transpose((1, 2, 0)), n_segments=n_segments) AttributeError: 'numpy.ndarray' object has no attribute 'numpy'
根据报错信息`AttributeError: 'numpy.ndarray' object has no attribute 'numpy'`,可以看出是因为尝试在numpy数组上调用numpy函数而导致的错误。具体来说,是因为在代码中使用了`image.numpy()`,而image是一个numpy数组,不需要再次调用numpy函数。
可以将以下代码:
```
segments = slic(image.numpy().transpose((1, 2, 0)), n_segments=n_segments)
```
修改为:
```
segments = slic(image.transpose((1, 2, 0)), n_segments=n_segments)
```
即可解决该问题。
阅读全文
相关推荐















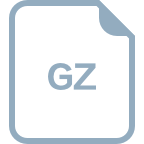
