上述代码报错all input arrays must have the same shap
时间: 2024-11-05 17:23:46 浏览: 6
这段代码中提到的错误 "all input arrays must have the same shape" 是因为在使用 `np.meshgrid` 或者 `np.stack` 时,输入的所有数组需要有相同的形状。这意味着所有传递给这些函数的数组在维度上应保持一致,以便进行正确的组合。
例如,在 `np.meshgrid` 中,对于每一个维度,所有的输入数组必须都具有相同的长度。如果其中有一个数组的长度与其他不同,就会抛出这个错误。同样地,当 `np.stack` 被用来拼接多维数组时,如果最后一维的大小不匹配,也可能会触发这个错误。
解决此问题,你需要确保 `x`, `y`, `z`, 和 `t` 都是具有相同长度的一维数组或者是形状完全匹配的二维数组,然后再进行操作。如果你想要保持每个维度的独立变化,可以考虑使用循环或者其他方式逐个处理每一维的范围。
```python
# 确保输入数组形状一致
if x.shape != y.shape or x.shape != z.shape or x.shape != t.shape:
raise ValueError("All input arrays must have the same shape.")
# 或者使用其他方式逐个生成网格
x, y, z = [np.linspace(0, 100, num=101) for _ in range(3)]
t = np.linspace(0, 100, num=101)
```
一旦解决了这个问题,你的代码就能正常运行并生成所需的四维数据 `points`。
相关问题
上面代码报错 ValueError: all the input arrays must have same number of dimensions
如果您遇到了 `ValueError: all the input arrays must have same number of dimensions` 错误,那么您可以尝试对标签进行维度变换,以使它们具有相同的维度。以下是一个示例代码,展示了如何将标签从一维数组转换为二维数组:
```python
import numpy as np
from sklearn.preprocessing import label_binarize
from sklearn.metrics import ndcg_score
# 生成样本标签
y_true = np.array([1, 2, 3, 4, 5])
y_pred = np.array([1, 1, 3, 2, 4])
# 将标签转换为 multiclass-multioutput 格式
classes = np.unique(np.concatenate((y_true,y_pred)))
y_true = label_binarize(y_true, classes=classes).reshape((-1, len(classes)))
y_pred = label_binarize(y_pred, classes=classes).reshape((-1, len(classes)))
# 计算 NDCG 值
ndcg_result = ndcg_score(y_true, y_pred, k=5)
print(ndcg_result)
```
在这个示例中,我们首先使用 `label_binarize` 函数将标签转换为 `multiclass-multioutput` 格式,然后使用 `reshape` 函数将它们的维度变换为相同的二维数组。最后,我们将转换后的标签传入到 `ndcg_score` 函数中计算 NDCG 值。
出现报错 All arrays must be of the same length
这个报错是因为读取的JSON文件中,不同的数据对象的长度不一致。如果使用`pd.read_json()`方法读取JSON文件时,没有指定`lines=True`参数,则默认将JSON文件中的每个对象当作一列数据,这就要求每个对象的长度必须相同。如果长度不同,则会报出"All arrays must be of the same length"的错误。
如果您的JSON文件中有多个数据对象,且这些数据对象的长度不一致,可以尝试将`lines=True`参数传递给`pd.read_json()`方法,这样会将每行数据解析为一个JSON对象,而不是将每个对象解析为一列数据。例如:
```python
import pandas as pd
# 读取JSON文件,将每行数据解析为JSON对象
data = pd.read_json('data.json', lines=True)
# 显示数据
print(data)
```
这样做可以避免"All arrays must be of the same length"的错误,也可以正确地读取JSON文件中的所有数据对象。
阅读全文
相关推荐
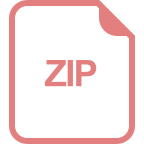
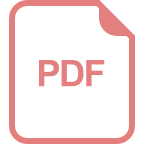
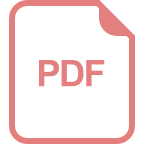


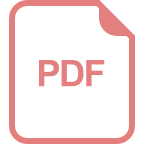
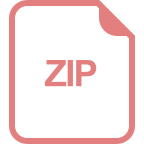
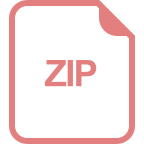
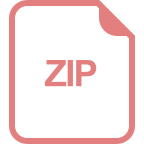
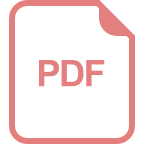
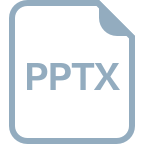
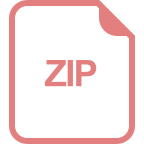
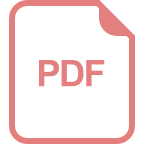
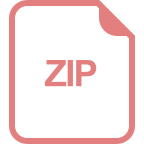
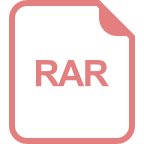