怎么把图片放进python中进行图片识别
时间: 2024-12-23 07:18:04 浏览: 9
在Python中进行图像识别,通常会利用深度学习库如TensorFlow、PyTorch或者开源的图像处理库PIL(Python Imaging Library)和OpenCV。以下是基本步骤:
1. **安装必要的库**:
- 对于机器学习库,比如TensorFlow可以使用`pip install tensorflow`,而PyTorch则是`pip install torch torchvision`。
- PIL用于基本的图像操作,例如读取和显示图片,`pip install pillow`即可。
2. **加载图片**:
使用PIL库的`Image.open()`函数打开图片文件,例如:
```python
from PIL import Image
image = Image.open('image.jpg')
```
3. **预处理图片**:
图像通常需要调整尺寸、颜色空间转换等以便输入到模型中。例如,对ResNet50这样的模型,一般要求图片统一为特定大小,并转换为RGB通道。
```python
from tensorflow.keras.applications.resnet50 import preprocess_input
image = image.resize((224, 224)) # 调整为模型所需的尺寸
image = np.array(image) / 255.0 # 归一化像素值
input_array = preprocess_input(image)
```
4. **使用预训练模型**:
TensorFlow或PyTorch提供了许多预训练的图像分类模型,如InceptionV3、ResNet等。你可以通过模型的`predict()`或`.forward()`方法进行预测:
```python
if using_tensorflow:
predictions = model.predict(np.expand_dims(input_array, axis=0))
elif using_pytorch:
with torch.no_grad():
prediction = model(torch.tensor(input_array).unsqueeze(0)).argmax(dim=1)
```
5. **解读结果**:
获取到的predictions是一个概率分布,最高概率的索引对应的类别就是模型认为图片属于的类别。
阅读全文
相关推荐










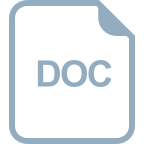


