编写python程序删除文件中的特殊字符,单行注释及回车换行符
时间: 2024-10-18 10:19:30 浏览: 28
在Python中,你可以使用内置模块`re`(正则表达式)来删除文件中的特殊字符、单行注释以及回车换行符。这里有一个简单的示例:
```python
import re
# 定义一个函数来清理文件内容
def clean_file_content(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read() # 读取文件内容
# 删除特殊字符(可以自定义需要保留的字符)
cleaned_content = re.sub(r'[^\w\s]', '', content)
# 删除单行注释(如以#开头的行)
cleaned_content = '\n'.join(line for line in cleaned_content.split('\n') if not line.startswith('#'))
# 删除所有多余的空白行和回车换行符
cleaned_content = re.sub(r'\n+', '\n', cleaned_content).strip()
return cleaned_content
# 使用函数并替换原文件
cleaned_content = clean_file_content('your_file.txt')
with open('your_file_cleaned.txt', 'w', encoding='utf-8') as file:
file.write(cleaned_content)
```
在这个例子中,我们首先读取文件内容,然后使用正则表达式删除所有非字母数字字符(特殊字符)。接着,我们通过列表推导式过滤掉以`#`开头的单行注释。最后,我们将内容格式化为只包含单个连续的新行。
阅读全文
相关推荐
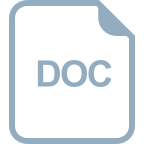
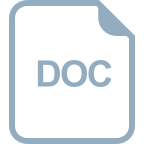
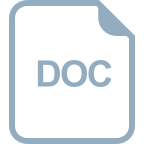

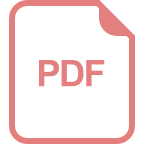
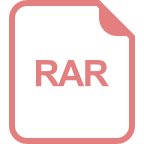
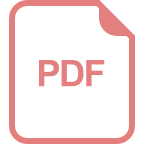






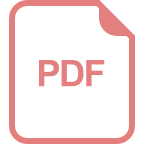
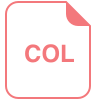



