适合初学者Java的代码
时间: 2024-12-02 08:19:07 浏览: 6
好的,我可以给你一些简单的Java代码示例,这些代码可以帮助初学者理解Java的基础概念。
1. **Hello World程序**:这是任何编程语言中最基本的程序。
```java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
```
2. **控制台输入输出**:使用Java控制台输入和输出。
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入你的名字:");
String name = scanner.nextLine();
System.out.println("你好," + name + "!");
}
}
```
3. **简单的计算器**:这是一个使用Java Swing库创建的简单图形用户界面(GUI)计算器程序。它可以进行加、减、乘、除运算。
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class SimpleCalculator extends JFrame {
private JTextField textField;
private JButton[] buttons;
private ButtonHandler buttonHandler;
public SimpleCalculator() {
setSize(400, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new FlowLayout());
setLocationRelativeTo(null); // Center the window on screen.
textField = new JTextField(); // for output to the user, say something! 1+2=... etc.. use "+=" if you want it to keep track of a variable, eg 1+= etc.. "5+=1=?" is another option to get people interested..
buttons = new JButton[9]; // Numbers and operation buttons...
buttonHandler = new ButtonHandler(); // event handler... or use a KeyListener for the numbers if you want to use the keyboard for input... for the operation buttons, you might want to use a MouseListener... or a combination of all three... you can also use a single KeyListener for the whole thing... just depends on what you want to do... and how much time you have.. :)
for (int i = 0; i < buttons.length; i++) { // set up the buttons... make them look like numbers... make them light up when clicked... make them darken when clicked again... use JLabel for this.. you can use setBackground for this.. you can also use JLabel's setIcon method to use images as buttons... for this example, I'll just use text... you can also use a Font object to change the font... you can also use FontMetrics to get the size of the button and adjust it accordingly... you can also use setSize for this.. you can also use setPreferredSize for this.. you can also use setMinimumSize for this.. and so on... :)
buttons[i] = new JButton("" + i); // make it look like a number... use JLabel to make it a label, and you can use it like any other label!
buttons[i].addActionListener(buttonHandler); // set up an action listener for each button... the button handler will be set up later..
add(buttons[i]); // add the button to the window..
}
add(textField); // add the text field for the user to enter numbers into and output the results from the calculator onto..
setVisible(true); // make it visible now..
}
private class ButtonHandler implements ActionListener { // handle button clicks...
public void actionPerformed(ActionEvent e) { // see if the button was clicked...
if (e.getSource() == buttons[1]) { // if they clicked one of the operation buttons...
String s = textField.getText(); // get the text from the text field into a string...
textField.setText(s + "="); // set the text back to "num=num+num" or whatever operation you want to perform...
} else { // if they clicked a number button...
int num = Integer.parseInt(e.getActionCommand()); // get the number from the button into an integer...
textField.setText(textField.getText() + num); // add the number to the text in the text field...
}
}
}
}
阅读全文
相关推荐
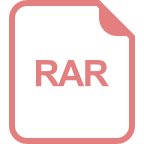
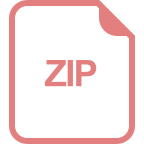
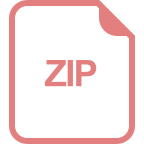
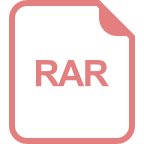
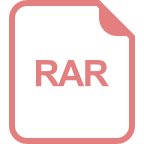
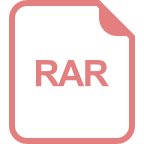
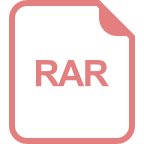
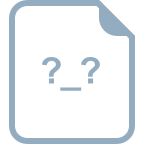
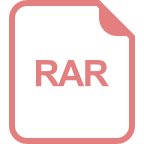
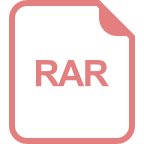
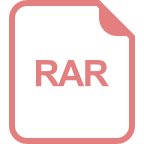
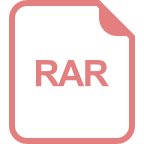
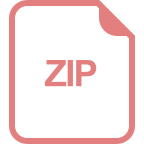
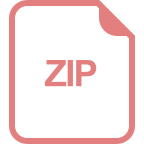
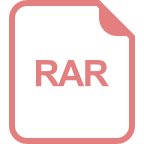