python烟火完全代码
时间: 2023-11-16 14:58:54 浏览: 47
以下是Python实现烟花效果的完整代码,包括了Tkinter库的使用和烟花效果的实现。你可以直接复制代码并运行,也可以根据自己的需求进行修改和优化。
```
import random
import tkinter as tk
class Particle:
def __init__(self, x, y, vx, vy, size, color):
self.x = x
self.y = y
self.vx = vx
self.vy = vy
self.size = size
self.color = color
def move(self):
self.x += self.vx
self.y += self.vy
self.vy += 0.1
def draw(self, canvas):
x1 = self.x - self.size
y1 = self.y - self.size
x2 = self.x + self.size
y2 = self.y + self.size
canvas.create_oval(x1, y1, x2, y2, fill=self.color, outline=self.color)
class Firework:
def __init__(self, x, y, canvas):
self.x = x
self.y = y
self.canvas = canvas
self.particles = []
self.color = random.choice(['red', 'green', 'blue', 'yellow', 'white'])
for i in range(50):
vx = random.uniform(-1, 1)
vy = random.uniform(-1, 1)
size = random.uniform(1, 3)
particle = Particle(x, y, vx, vy, size, self.color)
self.particles.append(particle)
def move(self):
for particle in self.particles:
particle.move()
def draw(self):
for particle in self.particles:
particle.draw(self.canvas)
class FireworkDisplay:
def __init__(self, width, height):
self.width = width
self.height = height
self.root = tk.Tk()
self.canvas = tk.Canvas(self.root, width=width, height=height)
self.canvas.pack()
self.fireworks = []
def launch_firework(self, event):
x = event.x
y = event.y
firework = Firework(x, y, self.canvas)
self.fireworks.append(firework)
def animate(self):
for firework in self.fireworks:
firework.move()
firework.draw()
self.root.after(10, self.animate)
def run(self):
self.canvas.bind('<Button-1>', self.launch_firework)
self.animate()
self.root.mainloop()
if __name__ == '__main__':
display = FireworkDisplay(800, 600)
display.run()
```
相关推荐
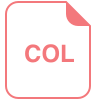
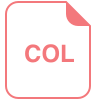
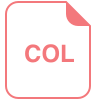
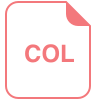
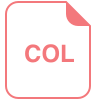










