springboot 事务配置
时间: 2023-11-08 13:55:26 浏览: 49
Spring Boot的事务配置非常简单。首先,使用注解@EnableTransactionManagement开启事务支持。然后,在访问数据库的Service方法上添加注解@Transactional即可。此外,关于事务管理器,如果你添加的是spring-boot-starter-jdbc依赖,框架会默认注入DataSourceTransactionManager实例;如果你添加的是spring-boot-starter-data-jpa依赖,框架会默认注入JpaTransactionManager实例。在Spring容器中,我们手工注解@Bean将被优先加载,框架不会重新实例化其他的PlatformTransactionManager实现类。
相关问题
springboot事务配置
在Spring Boot中,我们可以使用注解来配置事务。具体步骤如下:
1. 在主应用程序类上添加@EnableTransactionManagement注解启用事务管理。例如:
```
@SpringBootApplication
@EnableTransactionManagement
public class MyApp {
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
}
}
```
2. 在需要使用事务的方法上添加@Transactional注解。例如:
```
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Transactional
public void save(User user) {
userRepository.save(user);
}
}
```
3. 配置事务管理器。可以使用Spring Boot提供的默认事务管理器,也可以自定义事务管理器。例如:
```
@Configuration
@EnableTransactionManagement
public class AppConfig {
@Bean
public DataSource dataSource() {
// 配置数据源
}
@Bean
public PlatformTransactionManager transactionManager() {
return new DataSourceTransactionManager(dataSource());
}
}
```
以上就是Spring Boot中配置事务的基本步骤。需要注意的是,事务注解只对public方法有效,并且只有在Spring容器中才能生效。
springboot 事务配置实例
好的,下面是一个简单的 Spring Boot 事务配置实例:
1. 在 pom.xml 文件中添加如下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
```
2. 在 application.properties 文件中配置数据库连接信息:
```properties
spring.datasource.url=jdbc:mysql://localhost:3306/demo?useUnicode=true&characterEncoding=utf8&serverTimezone=UTC
spring.datasource.username=root
spring.datasource.password=root
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
# jpa配置
spring.jpa.show-sql=true
spring.jpa.hibernate.ddl-auto=update
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQL5InnoDBDialect
```
3. 创建一个 Service 类,在其中定义需要进行事务管理的方法:
```java
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
// 添加事务注解
@Transactional(rollbackFor = Exception.class)
public void addUser(User user) {
// ......
}
}
```
4. 在 Controller 类中调用 Service 中的方法:
```java
@RestController
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
@PostMapping("/add")
public String addUser(User user) {
userService.addUser(user);
return "success";
}
}
```
通过在 Service 中添加 `@Transactional` 注解,来开启事务管理。如果在方法执行过程中发生异常,则会回滚到事务开始前的状态。在 Controller 中调用 Service 方法时,如果出现异常,则会抛出 `RuntimeException` 异常,从而触发事务回滚。
相关推荐
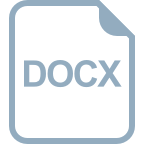












