请使用C/C++语言编程实现一种航路规划算法要求:(1)可任意选择起点和终点; (2) 可任意设置障碍点或区,航路可重新规划; (3) 绘制算法流程图及设计说明文档一份,功能录屏或可执行程序包一份
时间: 2024-10-13 11:10:08 浏览: 27
在C/C++中实现航路规划算法通常涉及到路径搜索技术,如A*搜索算法、Dijkstra算法或者RRT(Rapidly-exploring Random Tree)等。下面是一个简化的A*算法示例,用于在一个无阻碍的二维网格上找到两点之间的最短路径。请注意,实际应用中会增加对障碍物的处理,并可能使用图形库如SFML或Qt来绘制路径。
```cpp
#include <iostream>
#include <vector>
#include <queue>
struct Node {
int x, y;
int f, g, h; // f = g + h (启发式)
Node(int x_, int y_) : x(x_), y(y_), g(0), h(std::sqrt(std::pow(x - target_x, 2) + std::pow(y - target_y, 2))) {}
};
bool isWalkable(int i, int j, const std::vector<std::vector<int>>& obstacles) {
return obstacles[i][j] == 0;
}
std::vector<Node> AStarSearch(const int start_x, const int start_y,
const int target_x, const int target_y,
const std::vector<std::vector<int>>& obstacles) {
std::priority_queue<Node, std::vector<Node>, std::greater<Node>> open_list;
open_list.push({start_x, start_y});
std::vector<std::vector<bool>> came_from(grid_size, std::vector<bool>(grid_size, false));
std::vector<Node> path;
while (!open_list.empty()) {
Node current = open_list.top();
open_list.pop();
if (current.x == target_x && current.y == target_y) {
break;
}
for (const auto& neighbor : neighbors(current)) {
if (isWalkable(neighbor.x, neighbor.y, obstacles) &&
!came_from[neighbor.x][neighbor.y]) {
came_from[neighbor.x][neighbor.y] = true;
neighbor.f = current.g + 1;
open_list.push(neighbor);
}
}
}
// Reconstruct the path
if (current.x != target_x || current.y != target_y) {
return {}; // No path found
} else {
Node current_node = current;
while (current_node.x != start_x || current_node.y != start_y) {
path.push_back(current_node);
current_node = came_from[current_node.x][current_node.y];
}
path.push_back(Node(start_x, start_y)); // Add starting point to complete the path
std::reverse(path.begin(), path.end());
}
return path;
}
// ... 其他辅助函数如计算邻居节点等
int main() {
// 初始化网格大小,障碍点,起点和目标位置
// ...
std::vector<Node> planned_path = AStarSearch(start_x, start_y, target_x, target_y, obstacles);
// 使用path遍历并绘制路径,或者保存到文件或生成可执行程序
// ...
return 0;
}
```
为了满足所有需求,你需要编写详细的流程图描述每个步骤,包括初始化、状态更新、路径评估和决策等。设计说明文档应该涵盖算法的工作原理、数据结构的选择以及如何处理障碍物。录制功能录屏可能需要专业的屏幕录制软件,而可执行程序包的制作则依赖于构建工具如g++或Visual Studio。
阅读全文
相关推荐
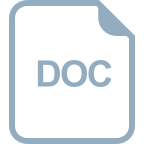
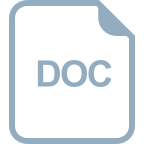
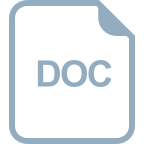
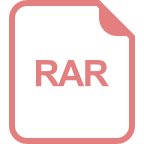
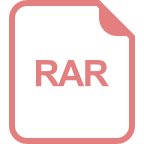
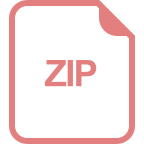
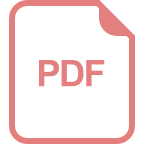
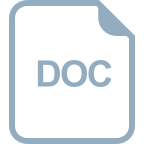
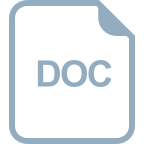
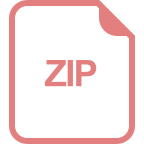
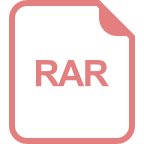
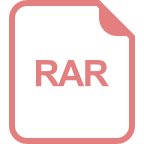
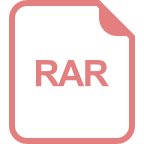
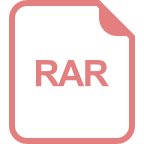
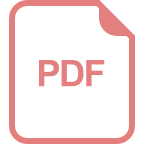
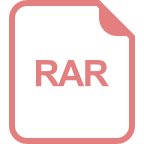
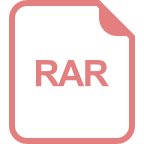
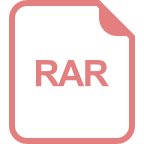