使用 Spring Boot 和 MyBatis 开发一个更改自编码的功能,判断输入的编码是属于表一还是表二,确保新编码在表一和表二中唯一,修改表一是同步表三和表四中跟表一一致的编码,不用判断重复
时间: 2024-03-28 17:42:36 浏览: 44
好的,我理解了你的要求。首先,你需要在 Spring Boot 中集成 MyBatis,并且配置两个数据源分别对应表一和表二。然后,你需要编写一个接口来实现更改自编码的功能。这个接口应该包括以下步骤:
1. 根据输入的编码,判断是属于表一还是表二;
2. 在对应的表中查询是否存在该编码,如果存在,直接返回错误提示;
3. 如果不存在,更新对应表的编码;
4. 如果是表一,同步更新表三和表四中跟表一一致的编码。
下面是一个简单的实现示例:
```java
@Service
public class CodeService {
@Autowired
private TableOneMapper tableOneMapper;
@Autowired
private TableTwoMapper tableTwoMapper;
@Autowired
private TableThreeMapper tableThreeMapper;
@Autowired
private TableFourMapper tableFourMapper;
@Transactional
public void updateCode(String code, String newCode) {
if (isTableOne(code)) {
// 判断表一中是否已存在该编码
if (tableOneMapper.findByCode(code) != null) {
throw new RuntimeException("编码已存在");
}
// 更新表一中的编码
tableOneMapper.updateCode(code, newCode);
// 同步更新表三和表四中与表一一致的编码
tableThreeMapper.updateCode(code, newCode);
tableFourMapper.updateCode(code, newCode);
} else {
// 判断表二中是否已存在该编码
if (tableTwoMapper.findByCode(code) != null) {
throw new RuntimeException("编码已存在");
}
// 更新表二中的编码
tableTwoMapper.updateCode(code, newCode);
}
}
private boolean isTableOne(String code) {
// 根据编码规则判断是属于表一还是表二
// 省略具体实现
}
}
```
在这个示例中,我们使用了 MyBatis 来操作数据库,其中 `TableOneMapper`、`TableTwoMapper`、`TableThreeMapper` 和 `TableFourMapper` 分别对应表一、表二、表三和表四的 Mapper。在更新表一的时候,我们使用了事务来保证数据的一致性。
阅读全文
相关推荐
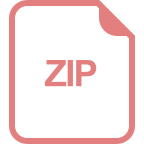
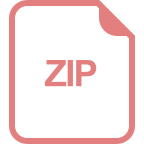


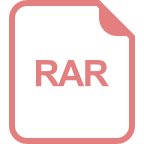
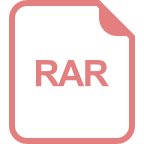
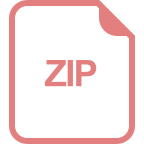
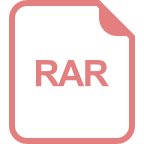
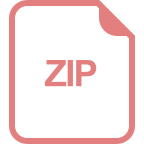
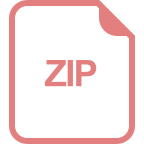
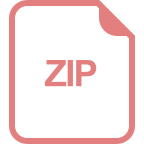
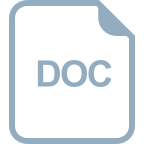
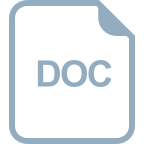
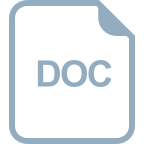
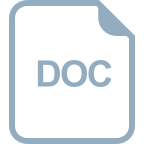
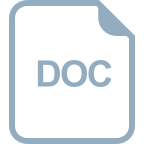
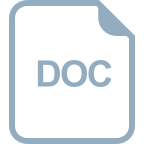
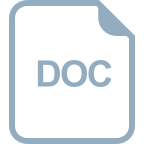