数字图像处理Python直接灰度变换增强对比度,并显示增强前后的图片和灰度直方图
时间: 2024-12-14 20:15:13 浏览: 3
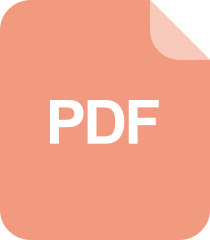
Python 图像对比度增强的几种方法(小结)
在Python中,数字图像处理通常通过一些库如PIL(Python Imaging Library)或其更现代的替代品OpenCV来进行。这里我们将展示如何使用PIL对一张彩色图片进行灰度转换,增强对比度,以及显示增强前后的图片和灰度直方图。
首先,你需要安装PIL库,如果还没有安装,可以使用pip安装:
```bash
pip install pillow
```
以下是步骤:
1. 导入所需的模块:
```python
from PIL import Image, ImageEnhance, ImageOps
import matplotlib.pyplot as plt
import numpy as np
```
2. 加载图片:
```python
img = Image.open('input_image.jpg')
```
3. 将图片转为灰度:
```python
gray_img = img.convert('L')
```
4. 创建对比度增强器并调整:
```python
enhancer = ImageEnhance.Contrast(gray_img)
contrast_img = enhancer.enhance(factor=1.5) # 调整对比度,factor大于1会增加对比度
```
5. 显示原图和增强后的灰度图像:
```python
fig, axs = plt.subplots(1, 2, figsize=(8, 4))
axs[0].imshow(gray_img, cmap='gray')
axs[0].set_title('Original Gray Image')
axs[1].imshow(contrast_img, cmap='gray')
axs[1].set_title('Contrast Enhanced Image')
plt.show()
```
6. 获取并显示灰度直方图:
```python
# 将灰度图像转换为numpy数组
gray_array = np.array(gray_img)
# 计算灰度直方图
hist, bins = np.histogram(gray_array.flatten(), bins=256, range=(0, 256))
# 绘制直方图
plt.figure(figsize=(10, 4))
plt.plot(bins[:-1], hist, color='blue', linewidth=2)
plt.title('Original Gray Histogram')
plt.xlabel('Gray Level')
plt.ylabel('Frequency')
plt.show()
# 对比增强后的直方图
hist_contrast, _ = np.histogram(np.array(contrast_img).flatten(), bins=256, range=(0, 256))
plt.figure()
plt.plot(bins[:-1], hist_contrast, color='red', linewidth=2)
plt.title('Contrast Enhanced Gray Histogram')
plt.xlabel('Gray Level')
plt.ylabel('Frequency')
plt.show()
```
这将展示原始灰度图像、增强对比度后的图像,以及两个灰度直方图,直观地比较了对比度变化前后的情况。
阅读全文
相关推荐
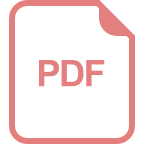
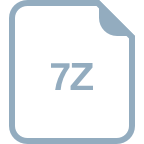
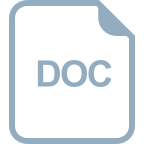
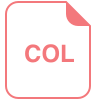


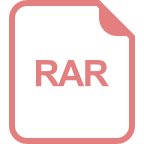
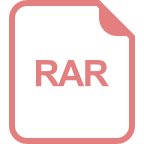
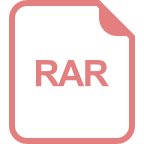
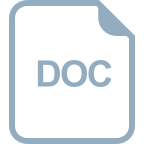
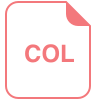
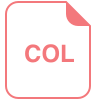

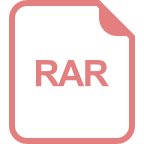
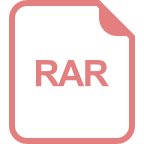
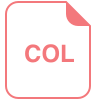

