编写Java程序,输入5名学生的5门课程的成绩,求其平均分和总分,然后再输出测试的平均分和最高分
时间: 2024-05-07 14:23:35 浏览: 134
以下是一个简单的 Java 程序,可以实现上述要求:
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int[][] scores = new int[5][5]; // 定义一个二维数组,存储5名学生的5门课程成绩
int[] studentTotal = new int[5]; // 定义一个数组,存储5名学生的总分
int[] testTotal = new int[5]; // 定义一个数组,存储5门课程的总分
// 输入每个学生每门课程的成绩,并计算总分和平均分
for (int i = 0; i < 5; i++) {
System.out.println("请输入第" + (i + 1) + "名学生的5门课程成绩:");
for (int j = 0; j < 5; j++) {
scores[i][j] = input.nextInt();
studentTotal[i] += scores[i][j];
testTotal[j] += scores[i][j];
}
}
// 计算每个学生的平均分和测试的平均分
double[] studentAvg = new double[5]; // 定义一个数组,存储5名学生的平均分
double[] testAvg = new double[5]; // 定义一个数组,存储5门课程的平均分
int studentMax = 0; // 存储最高分
double testAvgTotal = 0; // 存储测试的平均分
for (int i = 0; i < 5; i++) {
studentAvg[i] = studentTotal[i] / 5.0;
if (studentAvg[i] > studentAvg[studentMax]) {
studentMax = i;
}
testAvg[i] = testTotal[i] / 5.0;
testAvgTotal += testAvg[i];
}
testAvgTotal /= 5.0;
// 输出结果
System.out.println("每个学生的平均分为:");
for (int i = 0; i < 5; i++) {
System.out.printf("第%d名学生的平均分为%.2f\n", i + 1, studentAvg[i]);
}
System.out.println("每门课程的平均分为:");
for (int i = 0; i < 5; i++) {
System.out.printf("第%d门课程的平均分为%.2f\n", i + 1, testAvg[i]);
}
System.out.printf("测试的平均分为%.2f\n", testAvgTotal);
System.out.printf("最高分为%d分,是第%d名学生的成绩", scores[studentMax][0], studentMax + 1);
}
}
```
这个程序中,我们使用了一个二维数组 `scores` 来存储每个学生每门课程的成绩,一个一维数组 `studentTotal` 来存储每个学生的总分,一个一维数组 `testTotal` 来存储每门课程的总分。接着,我们使用两个循环,分别输入每个学生每门课程的成绩,并计算每个学生的总分和每门课程的总分。
接着,我们再使用一个循环,计算每个学生的平均分和每门课程的平均分,以及测试的平均分和最高分。最后,我们输出每个学生的平均分、每门课程的平均分、测试的平均分和最高分。
阅读全文
相关推荐
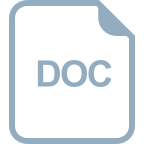
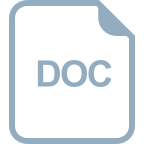
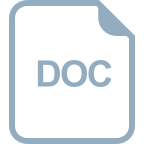
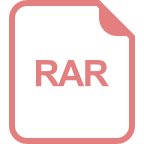
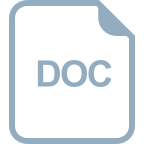
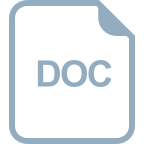
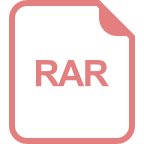
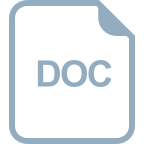
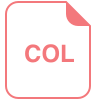
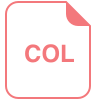
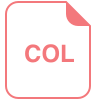
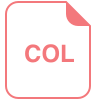
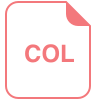
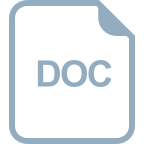
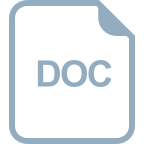
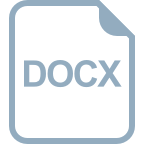
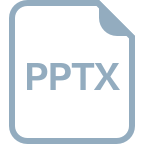
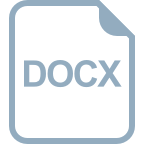