设计一个C++程序,统计元音字母(包括大小写)的个数,统计读入空格、制表符、换行符的个数,改写程序使其可以统计双字符序列ff、fl、fi的个数。
时间: 2024-10-19 12:04:25 浏览: 19
设计一个C++程序来统计元音字母(a, e, i, o, u,包括大写),空白字符(空格、制表符、换行符),以及特定的双字符序列(如ff, fl, fi)的个数,你可以按照以下步骤进行:
```cpp
#include <iostream>
#include <cctype> // 包含用于处理字符类型的头文件
#include <string>
int count_vowels(const std::string& str) {
int vowel_count = 0;
for (char c : str) {
if (std::isalpha(c) && (c == 'a' || c == 'e' || c == 'i' || c == 'o' || c == 'u')) {
vowel_count++;
}
}
return vowel_count;
}
int count_whitespace(const std::string& str) {
int whitespace_count = 0;
for (char c : str) {
if (ispunct(c)) { // 判断是否是标点符号
continue;
}
if (isspace(c)) { // 检查空格、制表符或换行符
whitespace_count++;
}
}
return whitespace_count;
}
int count_double_chars(const std::string& str) {
int double_char_count = 0;
for (size_t i = 0; i < str.length() - 1; ++i) {
if (str[i] == 'f' && str[i + 1] == 'f') {
double_char_count++;
} else if (str[i] == 'f' && (str[i + 1] == 'l' || str[i + 1] == 'i')) {
double_char_count++;
}
}
return double_char_count;
}
int main() {
std::string input;
std::cout << "请输入字符串: ";
getline(std::cin, input);
int total_stats[] = {count_vowels(input), count_whitespace(input), count_double_chars(input)};
std::cout << "元音字母个数: " << total_stats[0] << "\n";
std::cout << "空白字符个数: " << total_stats[1] << "\n";
std::cout << "双字符序列 ff, fl, fi 的个数: " << total_stats[2] << "\n";
return 0;
}
```
在这个程序中,我们首先定义了三个函数分别负责计算元音、空白字符和双字符序列的个数。然后在`main()`函数中从用户那里获取输入,并将结果打印出来。
阅读全文
相关推荐
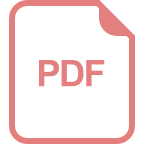
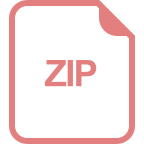
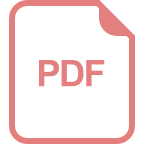
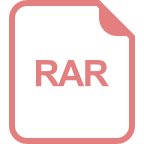
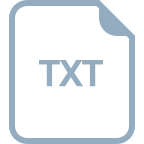
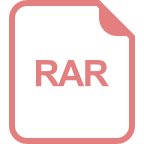
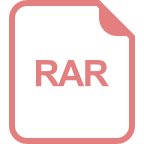
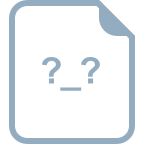
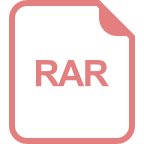
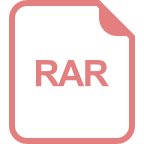
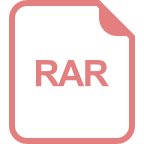
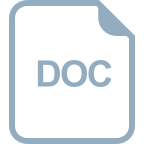
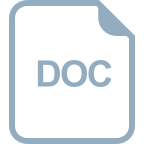