用java设计一个具有定时刷新或倒计时等时间功能,可根据需要设置按钮使其停止刷新时间;
时间: 2024-03-20 16:43:23 浏览: 14
好的,下面是一个简单的Java代码示例,演示如何设计一个具有定时刷新或倒计时等时间功能,以及如何设置按钮来停止刷新时间:
```java
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.*;
public class TimerExample extends JFrame implements ActionListener {
private Timer timer;
private JLabel label;
private JButton button;
public TimerExample() {
super("定时器示例");
label = new JLabel("倒计时:60秒");
button = new JButton("停止计时器");
button.addActionListener(this);
add(label, "North");
add(button, "South");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(300, 100);
setLocationRelativeTo(null);
setVisible(true);
// 创建计时器,每秒执行一次
timer = new Timer(1000, new ActionListener() {
int count = 60;
@Override
public void actionPerformed(ActionEvent e) {
count--;
label.setText("倒计时:" + count + "秒");
if (count == 0) {
timer.stop();
label.setText("时间到!");
}
}
});
timer.start();
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == button) {
timer.stop();
label.setText("计时器已停止!");
}
}
public static void main(String[] args) {
new TimerExample();
}
}
```
上述代码中,首先创建了一个JFrame窗口,包含一个JLabel标签和一个JButton按钮。然后创建了一个计时器Timer,每秒执行一次,并在计时器的ActionListener中实现倒计时功能。在按钮的ActionListener中调用Timer.stop方法来停止计时器。
你可以在自己的IDE中运行该代码,看看效果。当然,你也可以根据自己的需求修改代码,实现更加复杂的定时器功能。
相关推荐
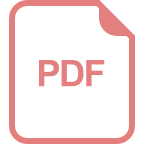
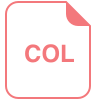













