用C语言编写文件复制程序。分别使用系统调用read (fd,buf,nbytes)、write(fd, buf,nbytes)和文件的库函数fread(buf,size,nitems,fp)、fwrite(buf,size,nitems,fp),编写一个文件复制程序
时间: 2024-02-03 10:14:47 浏览: 130
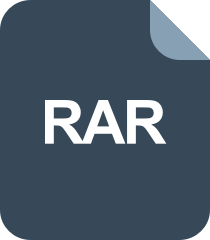
利用汇编写的文件操作小程序,功能是复制文件
使用系统调用read和write的文件复制程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#define BUFSIZE 1024
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("usage: %s <source> <destination>\n", argv[0]);
exit(EXIT_FAILURE);
}
int in_fd, out_fd;
char buffer[BUFSIZE];
ssize_t bytes_read;
// 打开源文件
in_fd = open(argv[1], O_RDONLY);
if (in_fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
// 创建目标文件
out_fd = open(argv[2], O_WRONLY | O_CREAT | O_TRUNC, S_IRUSR | S_IWUSR);
if (out_fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
// 读取源文件并写入目标文件
while ((bytes_read = read(in_fd, buffer, BUFSIZE)) > 0) {
if (write(out_fd, buffer, bytes_read) != bytes_read) {
perror("write");
exit(EXIT_FAILURE);
}
}
if (bytes_read == -1) {
perror("read");
exit(EXIT_FAILURE);
}
// 关闭文件
if (close(in_fd) == -1) {
perror("close");
exit(EXIT_FAILURE);
}
if (close(out_fd) == -1) {
perror("close");
exit(EXIT_FAILURE);
}
return 0;
}
```
使用文件的库函数fread和fwrite的文件复制程序:
```c
#include <stdio.h>
#include <stdlib.h>
#define BUFSIZE 1024
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("usage: %s <source> <destination>\n", argv[0]);
exit(EXIT_FAILURE);
}
FILE *in_fp, *out_fp;
char buffer[BUFSIZE];
size_t bytes_read;
// 打开源文件
in_fp = fopen(argv[1], "rb");
if (in_fp == NULL) {
perror("fopen");
exit(EXIT_FAILURE);
}
// 创建目标文件
out_fp = fopen(argv[2], "wb");
if (out_fp == NULL) {
perror("fopen");
exit(EXIT_FAILURE);
}
// 读取源文件并写入目标文件
while ((bytes_read = fread(buffer, 1, BUFSIZE, in_fp)) > 0) {
if (fwrite(buffer, 1, bytes_read, out_fp) != bytes_read) {
perror("fwrite");
exit(EXIT_FAILURE);
}
}
if (ferror(in_fp)) {
perror("fread");
exit(EXIT_FAILURE);
}
// 关闭文件
if (fclose(in_fp) != 0) {
perror("fclose");
exit(EXIT_FAILURE);
}
if (fclose(out_fp) != 0) {
perror("fclose");
exit(EXIT_FAILURE);
}
return 0;
}
```
阅读全文
相关推荐
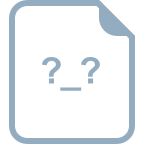






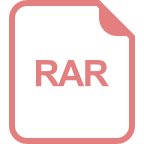
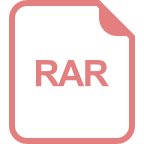
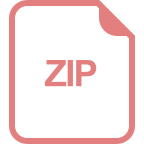