void countEducation() { unordered_map<string, int> bachelorCount, masterCount, doctoralCount; Node* p = head->next; while (p != nullptr) { string department = p->data.getDepartment(); string education = p->data.getEducation(); if (education == "本科") { bachelorCount[department]++; } else if (education == "硕士") { masterCount[department]++; } else if (education == "博士") { doctoralCount[department]++; } p = p->next; } for (auto it : bachelorCount) { cout << it.first << "本科:" << it.second << endl; } for (auto it : masterCount) { cout << it.first << "硕士:" << it.second << endl; } for (auto it : doctoralCount) { cout << it.first << "博士:" << it.second << endl; } }修改代码,使其输出时按照各个“系”对应输出各个学历的人数
时间: 2024-04-29 17:20:27 浏览: 70
void countEducation() {
unordered_map<string, unordered_map<string, int>> departmentCount;
Node* p = head->next;
while (p != nullptr) {
string department = p->data.getDepartment();
string education = p->data.getEducation();
departmentCount[department][education]++;
p = p->next;
}
for (auto it1 : departmentCount) {
cout << it1.first << ":\n";
for (auto it2 : it1.second) {
cout << " " << it2.first << ":" << it2.second << endl;
}
}
}
相关问题
翻译代码:void countEducation() { unordered_map<string, unordered_map<string, int>> departmentCount; Node* p = head->next; while (p != nullptr) { string department = p->data.getDepartment(); string education = p->data.getEducation(); departmentCount[department][education]++; p = p->next; } for (auto it1 : departmentCount) { cout << it1.first << ":\n"; for (auto it2 : it1.second) { cout << " " << it2.first << ":" << it2.second << endl; } } }
这段代码用于统计各个系中各个学历的人数。
函数定义为 `void countEducation()`,没有参数,没有返回值。
在函数内部,首先定义了一个 `unordered_map<string, unordered_map<string, int>>` 类型的变量 `departmentCount`,用于存储各个系中各个学历的人数。其中,外层的 `string` 类型作为键表示系名,内层的 `unordered_map` 类型的键为学历,值为人数。
接着,定义指针 `p` 指向链表的第一个元素,通过循环遍历整个链表。在每次循环中,获取当前节点的系名和学历,然后将该系名和学历对应的值加 1 存入 `departmentCount` 中。
最后,通过循环遍历 `departmentCount`,依次输出每个系的各个学历的人数。其中,外层循环遍历系名,内层循环遍历该系的各个学历及对应的人数。输出时,使用 `cout` 函数输出系名和学历对应的人数。
void countEducation() { Node* p = head->next; int bachelorCount = 0, masterCount = 0, doctoralCount = 0; while (p != nullptr) { if (p->data.getEducation() == "本科") { bachelorCount++; } else if (p->data.getEducation() == "硕士") { masterCount++; } else if (p->data.getEducation() == "博士") { doctoralCount++; } p = p->next; } cout << "本科:" << bachelorCount << endl; cout << "硕士:" << masterCount << endl; cout << "博士:" << doctoralCount << endl; 修改代码,只使用链表,使其可以分别按照“系”来统计各个系中的各种学历的人数 }
void countEducation() {
unordered_map<string, int> bachelorCount, masterCount, doctoralCount;
Node* p = head->next;
while (p != nullptr) {
string department = p->data.getDepartment();
string education = p->data.getEducation();
if (education == "本科") {
bachelorCount[department]++;
} else if (education == "硕士") {
masterCount[department]++;
} else if (education == "博士") {
doctoralCount[department]++;
}
p = p->next;
}
for (auto it : bachelorCount) {
cout << it.first << "本科:" << it.second << endl;
}
for (auto it : masterCount) {
cout << it.first << "硕士:" << it.second << endl;
}
for (auto it : doctoralCount) {
cout << it.first << "博士:" << it.second << endl;
}
}
修改后的代码中,使用了一个 unordered_map 来分别记录各个系中的本科、硕士和博士的人数。在遍历链表时,对于每个节点,根据其所属的系和学历,更新对应的计数器。最后,通过遍历三个 unordered_map,输出各个系中的各种学历的人数。
阅读全文
相关推荐
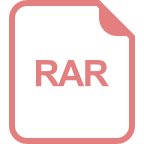
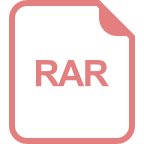
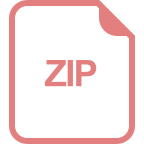
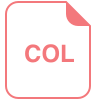
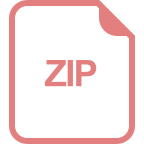
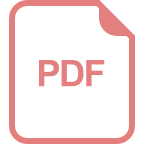
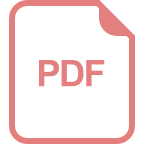
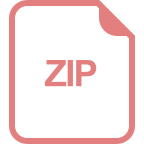
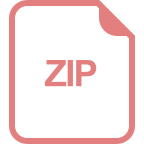
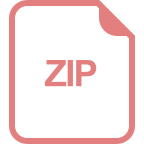
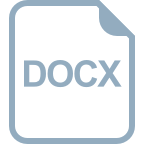
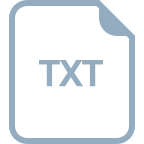
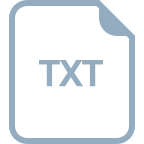