java龟兔赛跑图形界面
时间: 2023-09-22 20:08:34 浏览: 37
好的,以下是一个基于Java图形用户界面(GUI)的龟兔赛跑小程序。这个程序使用Java Swing库来创建GUI界面,还使用了多线程来模拟比赛。
```
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Random;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
public class TortoiseAndHareRaceGUI extends JFrame implements ActionListener, Runnable {
private static final long serialVersionUID = 1L;
private static final int WIDTH = 800;
private static final int HEIGHT = 600;
private static final int FINISH_LINE = 700;
private static final int TORTOISE_START_POSITION = 50;
private static final int HARE_START_POSITION = 50;
private static final int MOVE_PIXELS = 10;
private JPanel raceTrackPanel;
private JLabel tortoiseLabel;
private JLabel hareLabel;
private JButton startButton;
private Thread raceThread;
private int tortoisePosition;
private int harePosition;
private boolean raceInProgress;
public TortoiseAndHareRaceGUI() {
setTitle("Tortoise and Hare Race");
setSize(WIDTH, HEIGHT);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
tortoisePosition = TORTOISE_START_POSITION;
harePosition = HARE_START_POSITION;
raceInProgress = false;
setupGUI();
}
private void setupGUI() {
// Race track panel
raceTrackPanel = new JPanel() {
private static final long serialVersionUID = 1L;
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
// Draw starting line
g.setColor(Color.BLACK);
g.fillRect(0, 100, getWidth(), 5);
// Draw finishing line
g.setColor(Color.RED);
g.fillRect(FINISH_LINE, 0, 5, getHeight());
// Draw tortoise
g.setColor(Color.GREEN);
g.fillRect(tortoisePosition, 50, 50, 50);
// Draw hare
g.setColor(Color.BLUE);
g.fillRect(harePosition, 200, 50, 50);
}
};
raceTrackPanel.setLayout(new BorderLayout());
add(raceTrackPanel, BorderLayout.CENTER);
// Labels panel
JPanel labelsPanel = new JPanel(new GridLayout(2, 1));
tortoiseLabel = new JLabel("Tortoise: " + TORTOISE_START_POSITION);
tortoiseLabel.setFont(new Font("Arial", Font.BOLD, 20));
labelsPanel.add(tortoiseLabel);
hareLabel = new JLabel("Hare: " + HARE_START_POSITION);
hareLabel.setFont(new Font("Arial", Font.BOLD, 20));
labelsPanel.add(hareLabel);
add(labelsPanel, BorderLayout.NORTH);
// Start button
startButton = new JButton("Start");
startButton.addActionListener(this);
add(startButton, BorderLayout.SOUTH);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == startButton) {
if (!raceInProgress) {
startRace();
}
}
}
private void startRace() {
raceInProgress = true;
startButton.setEnabled(false);
raceThread = new Thread(this);
raceThread.start();
}
private void endRace() {
raceInProgress = false;
startButton.setEnabled(true);
// Determine winner
if (tortoisePosition >= FINISH_LINE && harePosition >= FINISH_LINE) {
setTitle("It's a tie!");
} else if (tortoisePosition >= FINISH_LINE) {
setTitle("Tortoise wins!");
} else {
setTitle("Hare wins!");
}
}
@Override
public void run() {
Random random = new Random();
while (tortoisePosition < FINISH_LINE && harePosition < FINISH_LINE) {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
// Tortoise's move
int tortoiseMove = random.nextInt(10) + 1;
if (tortoiseMove >= 1 && tortoiseMove <= 5) {
// Fast plod
tortoisePosition += MOVE_PIXELS * 3;
} else if (tortoiseMove >= 6 && tortoiseMove <= 7) {
// Slip
tortoisePosition -= MOVE_PIXELS * 6;
} else {
// Slow plod
tortoisePosition += MOVE_PIXELS;
}
if (tortoisePosition < 0) {
tortoisePosition = 0;
}
// Hare's move
int hareMove = random.nextInt(10) + 1;
if (hareMove >= 1 && hareMove <= 2) {
// Sleep
} else if (hareMove >= 3 && hareMove <= 4) {
// Big hop
harePosition += MOVE_PIXELS * 9;
} else if (hareMove == 5) {
// Big slip
harePosition -= MOVE_PIXELS * 12;
} else if (hareMove >= 6 && hareMove <= 8) {
// Small hop
harePosition += MOVE_PIXELS;
} else {
// Small slip
harePosition -= MOVE_PIXELS * 2;
}
if (harePosition < 0) {
harePosition = 0;
}
// Update labels
tortoiseLabel.setText("Tortoise: " + tortoisePosition);
hareLabel.setText("Hare: " + harePosition);
// Repaint race track
raceTrackPanel.repaint();
}
endRace();
}
public static void main(String[] args) {
TortoiseAndHareRaceGUI race = new TortoiseAndHareRaceGUI();
race.setVisible(true);
}
}
```
这个程序创建了一个GUI界面,包括一个赛道面板、一个标签面板和一个开始按钮。当用户点击开始按钮时,程序会启动一个模拟比赛的线程,并在赛道面板上绘制乌龟和兔子的移动轨迹。同时,程序还会更新标签面板上的比赛进程。当比赛结束时,程序会禁用开始按钮,并显示胜者的名字。
相关推荐






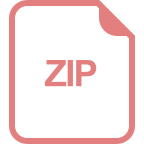
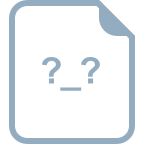
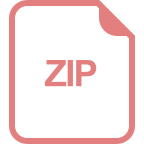
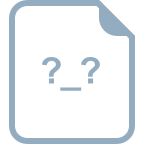
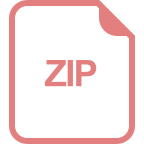