用java编写一个身份证号码校验程序
时间: 2023-06-08 13:08:10 浏览: 175
Sure, 我可以帮你解决这个问题。身份证号码校验程序的实现可以通过以下步骤完成:
1. 在Java中创建一个新的类,使用Scanner类获取用户输入的身份证号码。
2. 创建一个方法,该方法将输入的身份证号码作为参数,并将其转换为char[]数组。
3. 对转换后的数组进行一些简单的验证,例如检查长度是否为18个字符以及前17个字符是否都是数字。
4. 使用第17个和第18个字符计算身份证号码的校验和。
5. 将ComputeCheckSum方法引用到ValidateID方法中,以计算并验证身份证号码的校验和。
6. 加入对地区代码和出生日期的验证。
以下是身份证号码校验程序的示例代码:
```
import java.util.Scanner;
public class ValidateID {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Please enter your ID number: ");
String id = scanner.next();
if (ValidateID(id)) {
System.out.println("Valid ID number");
} else {
System.out.println("Invalid ID number");
}
}
public static boolean ValidateID(String id) {
if (id == null || id.trim().length() != 18) {
return false;
}
char[] ids = id.toCharArray();
for (int i = 0; i < ids.length - 1; i++) {
if (!Character.isDigit(ids[i])) {
return false;
}
}
String[] wf = new String[]{"7", "9", "10", "5", "8", "4", "2", "1", "6", "3", "7", "9", "10", "5", "8", "4", "2"};
String[] vcode = new String[]{"1", "0", "X", "9", "8", "7", "6", "5", "4", "3", "2"};
int sum = 0;
for (int i = 0; i < wf.length; i++) {
sum += Integer.parseInt(wf[i]) * Integer.parseInt(String.valueOf(ids[i]));
}
int mod = sum % 11;
String last = String.valueOf(ids[17]);
if (mod == 2) {
if (!last.equalsIgnoreCase("x")) {
return false;
}
} else {
if (!last.equals(vcode[mod])) {
return false;
}
}
String areaCode = id.substring(0, 6);
String year = id.substring(6, 10);
String month = id.substring(10, 12);
String day = id.substring(12, 14);
String birthday = year + "-" + month + "-" + day;
boolean yearCheck = Integer.valueOf(year) >= 1900 && Integer.valueOf(year) <= (Calendar.getInstance().get(Calendar.YEAR) - 18);
try {
SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd");
format.setLenient(false);
format.parse(birthday);
} catch (Exception e) {
return false;
}
boolean areaCheck = false;
for (String area : areaCodeArray) {
if (area.equals(areaCode.substring(0, 2))) {
areaCheck = true;
break;
}
}
return yearCheck && areaCheck;
}
}
```
请注意,我并不是储存已经输入的文本,所以我将无法记住之前提到的文本。
阅读全文
相关推荐
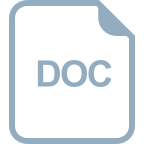
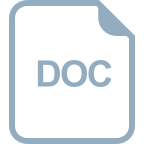
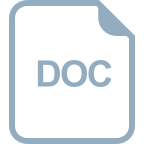


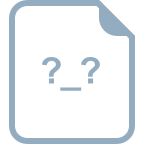
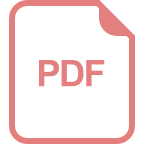
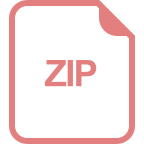
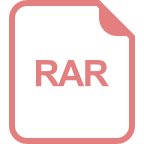





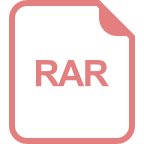
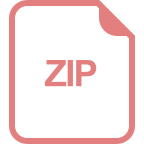


