pdf模板,java替换变量
时间: 2023-07-01 08:10:14 浏览: 64
您可以使用Apache PDFBox来读取和编辑PDF模板文件,并使用Java代码替换变量。
以下是一个简单的示例,演示如何在PDF模板中替换变量:
```java
import java.io.File;
import java.io.IOException;
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.pdmodel.PDPage;
import org.apache.pdfbox.pdmodel.edit.PDPageContentStream;
import org.apache.pdfbox.pdmodel.font.PDType1Font;
import org.apache.pdfbox.pdmodel.graphics.image.LosslessFactory;
import org.apache.pdfbox.pdmodel.graphics.image.PDImageXObject;
public class PDFEditor {
public static void main(String[] args) throws IOException {
// Load template PDF file
PDDocument document = PDDocument.load(new File("template.pdf"));
// Get the first page of the document
PDPage page = document.getPage(0);
// Create a new content stream to add text and images
PDPageContentStream contentStream = new PDPageContentStream(document, page, true, true);
// Set the font and font size for the text
contentStream.setFont(PDType1Font.HELVETICA_BOLD, 12);
// Replace the variable "name" with the actual name value
String name = "John Doe";
contentStream.beginText();
contentStream.newLineAtOffset(100, 700);
contentStream.showText(name);
contentStream.endText();
// Replace the variable "image" with the actual image file
PDImageXObject image = LosslessFactory.createFromImage(document, ImageIO.read(new File("image.jpg")));
contentStream.drawImage(image, 100, 600, image.getWidth(), image.getHeight());
// Close the content stream and save the document
contentStream.close();
document.save(new File("output.pdf"));
}
}
```
在此示例中,我们首先加载PDF模板文件,并获取第一页。然后,我们创建一个新的内容流,设置字体和字体大小,并使用`beginText()`和`endText()`方法添加文本。我们还使用`drawImage()`方法添加图像。
最后,我们关闭内容流并保存文档。在输出文件中,我们应该看到已经替换了模板中的变量。
请注意,这只是一个简单的示例,您可以根据自己的需求进行更改。
相关推荐
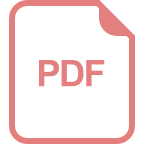














