micropython 两个线程都使用看门狗并设置超时为30秒,怎么实现,代码示例
时间: 2023-05-19 18:06:21 浏览: 64
可以使用以下代码实现:
import machine
import time
# 设置看门狗定时器
wdt = machine.WDT(timeout=30000)
# 线程1
def thread1():
while True:
# 线程1的任务
time.sleep(1)
# 喂狗
wdt.feed()
# 线程2
def thread2():
while True:
# 线程2的任务
time.sleep(1)
# 喂狗
wdt.feed()
# 启动线程1和线程2
import _thread
_thread.start_new_thread(thread1, ())
_thread.start_new_thread(thread2, ())
相关问题
micropython 两个线程都使用看门狗,怎么实现,代码示例
可以使用 MicroPython 的 threading 模块来实现两个线程都使用看门狗的功能。下面是一个简单的示例代码:
```python
import threading
import machine
def thread1():
while True:
# do something
machine.WDT.feed()
def thread2():
while True:
# do something
machine.WDT.feed()
# 创建两个线程
t1 = threading.Thread(target=thread1)
t2 = threading.Thread(target=thread2)
# 启动两个线程
t1.start()
t2.start()
```
在这个示例中,我们创建了两个线程 thread1 和 thread2,并在每个线程中使用 machine.WDT.feed() 函数来喂狗。这样,当任何一个线程出现问题时,看门狗都会在超时后重启设备。
需要注意的是,MicroPython 的看门狗定时器默认是关闭的,需要先使用 machine.WDT(timeout=5000) 函数来创建一个看门狗对象,并设置超时时间。在上面的示例中,我们假设超时时间为 5000 毫秒。
micropython 多个线程都使用同一个看门狗,怎么实现,代码示例
可以使用 MicroPython 的 Threading 模块来实现多个线程共享同一个看门狗。以下是一个示例代码:
```python
import machine
import time
import _thread
# 创建看门狗对象
wdt = machine.WDT()
# 定义线程函数
def thread_func():
while True:
# 线程执行的代码
print("Thread is running...")
time.sleep(1)
# 喂狗
wdt.feed()
# 创建两个线程并启动
_thread.start_new_thread(thread_func, ())
_thread.start_new_thread(thread_func, ())
```
在这个示例中,我们首先创建了一个看门狗对象 `wdt`,然后定义了一个线程函数 `thread_func`,该函数会不断地输出一条信息,并在每次循环结束时喂狗。最后,我们创建了两个线程并启动它们,这两个线程都会执行 `thread_func` 函数,并共享同一个看门狗对象。
需要注意的是,在使用看门狗时,我们需要定期喂狗,否则看门狗会认为系统出现了故障,从而触发复位。在这个示例中,我们使用 `time.sleep(1)` 函数来模拟线程执行的耗时操作,并在每次循环结束时喂狗。
相关推荐
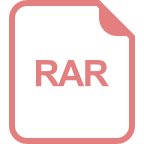
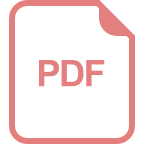
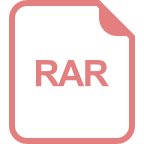












