gd32 自写modbus_rtu 从机功能
时间: 2023-08-28 22:02:41 浏览: 80
GD32是一款基于ARM Cortex-M系列核心的微控制器。通过自写Modbus RTU(Remote Terminal Unit)从机功能,可以实现与其他Modbus RTU主机或上位机之间的通信。
首先,Modbus是一种通信协议,用于在工业自动化系统中实现设备间的数据交互。RTU是Modbus协议的一种传输模式,通过串口通信传输数据。
自写Modbus RTU从机功能意味着我们在GD32微控制器上编写代码实现Modbus RTU协议的从机部分。这样,GD32就可以作为从机设备,参与到Modbus RTU网络中,与主机或上位机进行数据交换。
实际上,自写Modbus RTU从机功能需要编写适配GD32的Modbus协议栈代码。通过开发这样的代码,GD32可以解析和处理Modbus RTU主机或上位机发送的请求。作为从机设备,GD32可以提供数据读取、写入和控制等功能,以响应主机的请求。
通过自写Modbus RTU从机功能,GD32可以与其他Modbus RTU设备实现数据交互。例如,GD32作为一个传感器从机设备,可以提供环境温度、湿度等数据给主机设备;GD32作为一个执行器从机设备,可以接收主机的控制命令,执行相应的动作。
总结来说,通过GD32自写Modbus RTU从机功能,我们可以利用GD32的硬件资源和自主开发的Modbus协议栈代码,将GD32作为从机设备融入到Modbus RTU网络中,实现与主机或上位机之间的数据交换。
相关问题
gd32 gd32f4xx_usb_hw
gd32f4xx_usb_hw是基于GD32系列微控制器的USB硬件驱动库。
GD32系列是经过优化的RISC微控制器,具有高性价比和丰富的外设资源,适用于广泛的应用领域。gd32f4xx_usb_hw库是为了支持GD32系列微控制器的USB功能而开发的,它实现了USB硬件驱动,使GD32微控制器能够通过USB接口与外部设备进行通信。
gd32f4xx_usb_hw库提供了一套API接口,开发人员可以使用这些接口来配置和控制GD32微控制器的USB功能。通过这些接口,我们可以实现USB设备功能,如USB设备的连接、断开、配置等。同时,gd32f4xx_usb_hw库还提供了USB中断处理函数,用于处理USB传输过程中的中断事件。
通过gd32f4xx_usb_hw库,我们可以轻松地实现各种USB设备功能,如USB存储设备、USB音频设备、USB HID设备等。开发人员只需按照库提供的接口进行配置和编程,即可实现所需的USB功能。
总之,gd32f4xx_usb_hw库是为了方便开发人员在GD32系列微控制器上实现USB功能而设计的。它提供了一套易于使用的接口,使开发人员能够快速、简便地开发出基于USB的应用程序。
gd32 modbus
GD32 Modbus是一种基于GD32微控制器的Modbus通信协议。GD32系列微控制器是由国内厂商设计的一种高性能、低功耗的微控制器产品,具有丰富的外设接口和灵活的应用特性。Modbus是一种常用的工业通信协议,用于实现不同设备之间的通信和数据交换。
GD32 Modbus将GD32微控制器和Modbus通信协议相结合,可以实现GD32微控制器与其他Modbus设备之间的数据通信。通过GD32 Modbus,可以实现设备之间的数据采集、监控、控制等功能,适用于各种工业自动化、智能控制领域。
GD32 Modbus可以通过串口、以太网等方式进行通信,适用于不同的通信环境和应用场景。它还支持不同的Modbus通信方式,包括RTU、ASCII和TCP/IP等,能够满足不同设备的通信需求。
GD32 Modbus具有良好的稳定性和可靠性,能够确保设备之间的数据通信安全和稳定。同时,GD32 Modbus的应用开发也相对简单,配套有丰富的开发工具和文档,开发人员可以快速上手并实现需要的功能。
总之,GD32 Modbus是一种功能丰富、稳定可靠的Modbus通信解决方案,可以帮助工程师们实现设备之间的数据通信和控制,为工业自动化和智能控制领域的应用提供了便利。
相关推荐
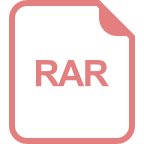
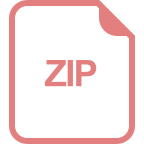












