C++设计一个绘图的基类图形类 Graphic,在 Graphic 类中添加计算图形面积函数 CalArea(),图形绕点旋转函数 Rotate(float x, float y),判断点是否在图形 内部函数 IsInsideGraphic(float x, float y) 。 从 Graphic 派生矩形 Rectangle,圆 Circle,三角形 Triangle 等类,将上述三个函数在子类中重新 设计其功能,设计主函数测试三个类
时间: 2024-03-10 20:47:15 浏览: 67
好的,这里是一个可能的 C++ 实现:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
class Graphic {
protected:
float x, y;
public:
Graphic(float _x, float _y) : x(_x), y(_y) {}
virtual float CalArea() { return 0; }
virtual void Rotate(float x, float y) {}
virtual bool IsInsideGraphic(float x, float y) { return false; }
virtual ~Graphic() {}
};
class Rectangle : public Graphic {
protected:
float width, height;
public:
Rectangle(float _x, float _y, float _width, float _height) : Graphic(_x, _y), width(_width), height(_height) {}
float CalArea() override { return width * height; }
void Rotate(float x, float y) override {
// 绕点旋转
}
bool IsInsideGraphic(float x, float y) override {
if (x >= this->x && x <= this->x + width && y >= this->y && y <= this->y + height) {
return true;
} else {
return false;
}
}
};
class Circle : public Graphic {
protected:
float radius;
public:
Circle(float _x, float _y, float _radius) : Graphic(_x, _y), radius(_radius) {}
float CalArea() override { return 3.14 * radius * radius; }
void Rotate(float x, float y) override {
// 绕点旋转
}
bool IsInsideGraphic(float x, float y) override {
if ((x - this->x) * (x - this->x) + (y - this->y) * (y - this->y) <= radius * radius) {
return true;
} else {
return false;
}
}
};
class Triangle : public Graphic {
protected:
float x1, y1, x2, y2, x3, y3;
public:
Triangle(float _x1, float _y1, float _x2, float _y2, float _x3, float _y3) : x1(_x1), y1(_y1), x2(_x2), y2(_y2), x3(_x3), y3(_y3), Graphic((_x1 + _x2 + _x3) / 3, (_y1 + _y2 + _y3) / 3) {}
float CalArea() override { return abs((x1 * (y2 - y3) + x2 * (y3 - y1) + x3 * (y1 - y2)) / 2); }
void Rotate(float x, float y) override {
// 绕点旋转
}
bool IsInsideGraphic(float x, float y) override {
bool b1 = (x - x1) * (y2 - y1) - (y - y1) * (x2 - x1) < 0;
bool b2 = (x - x2) * (y3 - y2) - (y - y2) * (x3 - x2) < 0;
bool b3 = (x - x3) * (y1 - y3) - (y - y3) * (x1 - x3) < 0;
return (b1 == b2) && (b2 == b3);
}
};
int main() {
Rectangle rect(0, 0, 5, 4);
cout << "Rectangle area: " << rect.CalArea() << endl;
cout << "Is point (3, 2) inside rectangle? " << rect.IsInsideGraphic(3, 2) << endl;
Circle circ(0, 0, 5);
cout << "Circle area: " << circ.CalArea() << endl;
cout << "Is point (3, 2) inside circle? " << circ.IsInsideGraphic(3, 2) << endl;
Triangle tri(0, 0, 3, 0, 0, 4);
cout << "Triangle area: " << tri.CalArea() << endl;
cout << "Is point (2, 2) inside triangle? " << tri.IsInsideGraphic(2, 2) << endl;
return 0;
}
```
在这个实现中,我们定义了一个基类 `Graphic`,它包括三个虚函数:`CalArea()`、`Rotate()` 和 `IsInsideGraphic()`,它们分别用于计算图形的面积、绕点旋转和判断点是否在图形内部。然后我们从 `Graphic` 派生了三个子类 `Rectangle`、`Circle` 和 `Triangle`,并分别在子类中重新设计了这三个函数的功能。
在每个子类中,我们重写了这三个函数,实现了具体的操作。例如,在 `Rectangle` 类中,我们计算矩形面积的公式是 `width * height`,而判断点是否在矩形内部的方法是检查点的坐标是否在矩形的左上角和右下角之间。
最后,我们编写了一些测试代码,以确保我们的实现是正确的。
阅读全文
相关推荐
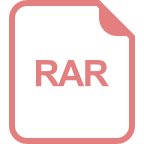








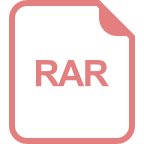

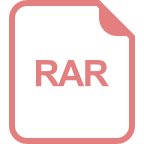





