python对用户发微博的日期进行统计并绘制柱状图
时间: 2024-05-02 10:23:09 浏览: 56
要对用户发微博的日期进行统计,需要先获取微博数据,并解析出每条微博的发布日期。可以使用Python的第三方库Tweepy来获取用户的微博数据,具体步骤如下:
1. 安装Tweepy库
```
pip install tweepy
```
2. 创建Twitter开发者账号,创建应用并获取API key和API secret
3. 使用Tweepy的OAuthHandler类进行认证并获取授权
```python
import tweepy
consumer_key = 'YOUR_CONSUMER_KEY'
consumer_secret = 'YOUR_CONSUMER_SECRET'
access_token = 'YOUR_ACCESS_TOKEN'
access_token_secret = 'YOUR_ACCESS_TOKEN_SECRET'
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth)
```
4. 使用Tweepy的API类获取用户的微博数据
```python
tweets = api.user_timeline(screen_name='USER_SCREEN_NAME', count=200, tweet_mode='extended')
for tweet in tweets:
created_at = tweet.created_at.date() # 获取微博发布日期
print(created_at)
```
5. 使用Python的第三方库matplotlib绘制柱状图
```python
import matplotlib.pyplot as plt
from collections import Counter
dates = [tweet.created_at.date() for tweet in tweets] # 获取所有微博的发布日期
date_counter = Counter(dates) # 统计每个日期的微博数量
x = list(date_counter.keys())
y = list(date_counter.values())
plt.bar(x, y)
plt.title('User Tweet Count by Date')
plt.xlabel('Date')
plt.ylabel('Tweet Count')
plt.show()
```
完整代码如下:
```python
import tweepy
import matplotlib.pyplot as plt
from collections import Counter
consumer_key = 'YOUR_CONSUMER_KEY'
consumer_secret = 'YOUR_CONSUMER_SECRET'
access_token = 'YOUR_ACCESS_TOKEN'
access_token_secret = 'YOUR_ACCESS_TOKEN_SECRET'
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth)
tweets = api.user_timeline(screen_name='USER_SCREEN_NAME', count=200, tweet_mode='extended')
dates = [tweet.created_at.date() for tweet in tweets]
date_counter = Counter(dates)
x = list(date_counter.keys())
y = list(date_counter.values())
plt.bar(x, y)
plt.title('User Tweet Count by Date')
plt.xlabel('Date')
plt.ylabel('Tweet Count')
plt.show()
```
阅读全文
相关推荐
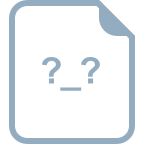
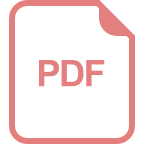
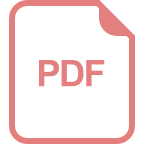















