python环境下有哪些多进程调度器
时间: 2023-05-20 17:02:47 浏览: 96
在Python环境下,有多个多进程调度器可供选择,包括:
1. multiprocessing模块:Python标准库中的多进程模块,提供了Process、Pool等类,可以方便地创建和管理多个进程。
2. concurrent.futures模块:Python标准库中的异步编程模块,提供了ThreadPoolExecutor和ProcessPoolExecutor两个类,可以方便地创建和管理线程池和进程池。
3. Celery:一个基于Python的分布式任务队列,可以用于异步处理任务,支持多进程、多线程和协程等方式。
4. Dask:一个基于Python的并行计算框架,支持多进程、多线程和分布式计算,可以用于处理大规模数据集。
5. Ray:一个基于Python的分布式计算框架,支持多进程、多线程和分布式计算,可以用于处理机器学习、深度学习等任务。
需要注意的是,选择哪个多进程调度器取决于具体的应用场景和需求,需要根据实际情况进行选择。
相关问题
python进程调度
Python进程调度是指在Python程序中,对多个进程进行合理的调度和分配CPU资源,以达到最优的执行效果。常见的进程调度算法有多级反馈队列调度算法、先来先服务调度算法、最短作业优先调度算法等。其中,多级反馈队列调度算法是一种较好的进程调度算法,它不必事先知道各种进程所需的执行时间,而且还可以满足各种类型进程的需要。在Python程序中,可以通过创建一个PCB类来表示进程,该类的属性包括进程序号id、状态state、开始执行时间starttime、执行结束时间endtime、所需执行时间cputime、剩余执行时间restoftime、已运行时间runtime等。通过对进程的状态进行判断和调度,可以实现Python程序的高效执行。
python进程调度算法
Python是一种高级编程语言,它本身并不负责进程调度。进程调度是操作系统的任务,各个操作系统有不同的进程调度算法。
常见的进程调度算法有以下几种:
1. 先来先服务调度算法(FCFS)
2. 最短作业优先调度算法(SJF)
3. 优先级调度算法
4. 时间片轮转调度算法
5. 多级反馈队列调度算法
Python的多线程和多进程模块(threading和multiprocessing)可以利用操作系统提供的进程调度算法实现并发执行。例如,在多进程模块中,可以使用Process类创建进程,然后由操作系统进行调度。在多线程模块中,可以使用Thread类创建线程,然后由操作系统进行调度。
需要注意的是,Python的GIL(全局解释器锁)会限制多线程的并发性能,因此在需要高并发处理的场景下,应该考虑使用多进程模块。
相关推荐
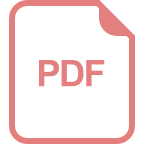
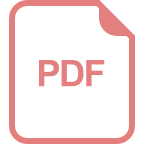
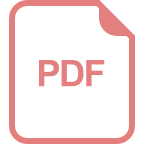










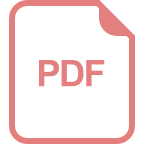
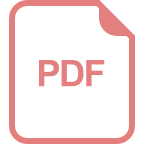
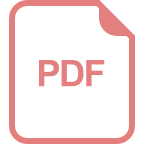