python re.sub替换多行数据
时间: 2023-07-26 09:04:06 浏览: 51
可以使用re.DOTALL标志来匹配多行数据,并使用re.sub函数进行替换。以下是一个例子:
```python
import re
text = '''
This is line 1.
This is line 2.
This is line 3.
'''
# 使用re.DOTALL标志匹配多行数据
pattern = re.compile('This is line 2.(.*?)(?=This is line|$)', re.DOTALL)
# 替换多行数据
new_text = re.sub(pattern, 'This is a new line.', text)
print(new_text)
```
输出:
```
This is line 1.
This is a new line.
This is line 3.
```
在上面的例子中,使用正则表达式匹配"This is line 2."和"This is line"之间的多行数据,并将其替换为"This is a new line."。注意,这里使用了零宽正向先行断言(?=This is line|$)来匹配"This is line"或字符串结尾,以避免替换掉最后一行数据。
相关问题
python根据字符串删除多行数据
可以使用Python中的字符串操作和正则表达式来删除多行数据。以下是一个例子:
```python
import re
text = """
This is the first line.
This is the second line.
This is the third line.
This is the fourth line.
This is the fifth line.
"""
# 删除第二行到第四行
text = re.sub(r"This is the second line.\nThis is the third line.\nThis is the fourth line.\n", "", text)
print(text)
```
输出结果为:
```
This is the first line.
This is the fifth line.
```
其中,`re.sub()` 函数使用正则表达式查找并替换匹配的文本。在这个例子中,我们使用正则表达式 `r"This is the second line.\nThis is the third line.\nThis is the fourth line.\n"` 来匹配想要删除的行。`\n` 表示换行符。
你也可以使用其他的正则表达式来匹配不同的行。如果你想要删除多个不连续的行,可以使用多次调用 `re.sub()` 函数。
python替换文件多行内容
Python 中替换文件多行内容可以通过以下步骤实现:
1. 打开文件并读取所有内容
2. 使用正则表达式或字符串函数替换需要替换的内容
3. 将修改后的内容写回文件中
下面是一个示例代码,假设我们需要将文件中的所有 "old_string" 替换为 "new_string",并且这个字符串可能出现在多行中:
```python
import re
# 打开文件并读取所有内容
with open('file.txt', 'r') as f:
content = f.read()
# 使用正则表达式替换多行字符串
new_content = re.sub(r'old_string', r'new_string', content, flags=re.MULTILINE)
# 将修改后的内容写回文件中
with open('file.txt', 'w') as f:
f.write(new_content)
```
上述代码中使用 `re.sub()` 函数进行正则表达式替换,其中 `flags=re.MULTILINE` 参数指定了多行模式,使得正则表达式中的 `^` 和 `$` 符号可以匹配每一行的开头和结尾。如果要替换的字符串不涉及正则表达式,可以使用 Python 的字符串函数 `replace()` 进行替换。
相关推荐
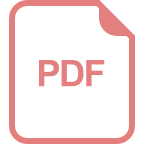
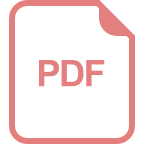
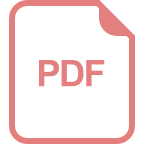












