c++通过进程名称判断是否存在
时间: 2024-03-20 14:41:29 浏览: 11
在C++中,可以通过操作系统提供的API来判断是否存在指定名称的进程。常用的方法是遍历系统中所有进程,查找指定名称的进程是否存在。
具体实现步骤如下:
1. 使用操作系统提供的API获取系统中所有进程的ID。
2. 遍历所有进程ID,通过操作系统提供的API获取每个进程的名称。
3. 判断每个进程名称是否与指定名称相同,如果相同,则表示指定名称的进程存在,否则不存在。
以下是一个示例代码,使用ps命令来获取进程名称:
```cpp
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
bool is_process_exist(const char* proc_name) {
FILE* fp;
char buf[1024];
char cmd[1024];
sprintf(cmd, "ps -ef | grep %s | grep -v grep", proc_name);
if ((fp = popen(cmd, "r")) != NULL) {
while (fgets(buf, 1024, fp) != NULL) {
if (strstr(buf, proc_name) != NULL) {
pclose(fp);
return true;
}
}
pclose(fp);
}
return false;
}
int main() {
const char* proc_name = "process_name"; // 指定进程名称
bool exist = is_process_exist(proc_name);
if (exist) {
printf("Process %s exists.\n", proc_name);
} else {
printf("Process %s does not exist.\n", proc_name);
}
return 0;
}
```
注意:在使用ps命令获取进程名称时,需要注意进程名称的匹配方式。如果进程名称中存在空格等特殊字符,需要进行相应的处理。另外,使用ps命令获取进程名称可能存在安全隐患,建议在实际使用中谨慎考虑。
相关推荐
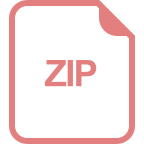
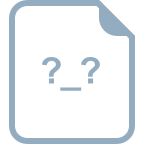









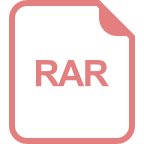
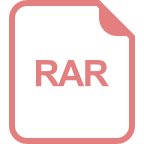
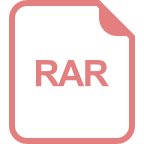
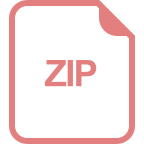
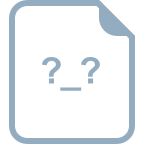