c++编程子串判断问题算法(设计) 有两个整数序列A=(a1,a2,a3……am)和B=(b1,b2,b3,……bn)将其存入两个单链表中,设计算法判断序列B是否在序列A中
时间: 2024-09-28 17:08:34 浏览: 19
在C++中,判断一个整数序列B是否包含在另一个整数序列A中,可以采用双指针法。这里是一个基本的设计思路:
1. 首先,你需要创建一个结构体或者类`Node`来表示链表中的节点,包含整数值和指向下一个节点的指针。
```cpp
struct Node {
int val;
Node* next;
};
```
2. 创建两个函数,`buildList`用于构建输入的整数序列到链表,`containsSubsequence`用于查找子序列B是否存在于A。
```cpp
// 构建链表
Node* buildList(vector<int>& nums) {
if (nums.empty()) return nullptr;
Node* head = new Node{nums[0], nullptr};
Node* tail = head;
for (int num : nums) {
tail->next = new Node{num, nullptr};
tail = tail->next;
}
return head;
}
// 判断子序列是否存在
bool containsSubsequence(Node* A, Node* B, int n) {
if (!A || !B || A->val != B->val) return false; // 检查起始值
Node* A_ptr = A;
Node* B_ptr = B;
while (n--) { // 遍历B链表
if (!A_ptr || A_ptr->val != B_ptr->val) break; // 如果A遍历完还没找到对应值,说明不存在
A_ptr = A_ptr->next;
B_ptr = B_ptr->next;
}
// 如果B遍历完了,说明找到了完整的子序列
return !B_ptr;
}
```
在这个算法中,我们首先比较两个链表的头节点,如果匹配则同时移动A和B的指针。如果不匹配,则检查A链表是否有剩余元素继续匹配。如果B链表遍历完还没有结束,那么B不在A中;反之,B就在A中。
相关推荐
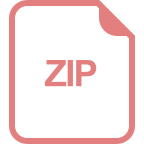
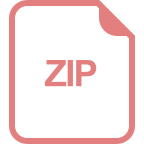
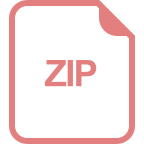














