vue+springboot怎么实现关注和取消关注的功能代码
时间: 2023-11-11 09:07:19 浏览: 143
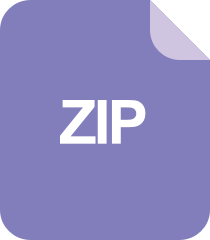
酒店管理系统(vue+springboot).zip
实现关注和取消关注的功能一般需要后端服务器提供相应的接口,以下是一个简单的实现方式。
假设我们有两个实体类:User(用户)和Follow(关注),User 包含用户的信息,Follow 则包含关注关系的信息。其中,User 实体类中有一个属性为 id,表示用户的唯一标识;Follow 实体类中有两个属性,一个为 followerId,表示关注者的 id,另一个为 followingId,表示被关注者的 id。
1. 添加关注
前端调用后端接口,将当前用户的 id 和被关注用户的 id 传递给后端,后端通过判断当前用户是否已经关注过被关注用户,如果已经关注则返回已关注的提示,否则插入一条 Follow 记录。
Java 代码实现:
```java
@PostMapping("/follow/{followingId}")
public ResponseEntity<?> follow(@PathVariable("followingId") Long followingId, Principal principal) {
User currentUser = userService.findByUsername(principal.getName());
User followingUser = userService.findById(followingId);
if (followingUser == null) {
return ResponseEntity.badRequest().body("Invalid followingId.");
}
Follow follow = followService.findByFollowerIdAndFollowingId(currentUser.getId(), followingId);
if (follow != null) {
return ResponseEntity.badRequest().body("You have already followed this user.");
}
follow = new Follow();
follow.setFollower(currentUser);
follow.setFollowing(followingUser);
followService.save(follow);
return ResponseEntity.ok().build();
}
```
2. 取消关注
前端调用后端接口,将当前用户的 id 和被取消关注用户的 id 传递给后端,后端通过判断当前用户是否已经关注过被关注用户,如果已经关注则删除对应的 Follow 记录,否则返回未关注的提示。
Java 代码实现:
```java
@DeleteMapping("/follow/{followingId}")
public ResponseEntity<?> unfollow(@PathVariable("followingId") Long followingId, Principal principal) {
User currentUser = userService.findByUsername(principal.getName());
User followingUser = userService.findById(followingId);
if (followingUser == null) {
return ResponseEntity.badRequest().body("Invalid followingId.");
}
Follow follow = followService.findByFollowerIdAndFollowingId(currentUser.getId(), followingId);
if (follow == null) {
return ResponseEntity.badRequest().body("You have not followed this user yet.");
}
followService.delete(follow);
return ResponseEntity.ok().build();
}
```
以上代码只是示例,实际应用中还需要对输入参数进行校验和安全性检查,保证系统的健壮性和安全性。
阅读全文
相关推荐
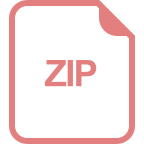
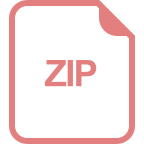















