使用Vue制作商品报表页面
时间: 2024-02-25 11:53:09 浏览: 124
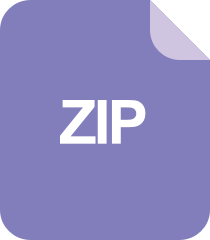
主页:使用Vue.js制作
使用 Vue 制作商品报表页面的具体步骤如下:
1. 在命令行中使用 Vue CLI 创建一个新的项目。
```
vue create report
```
2. 安装需要使用的 Vue 插件和第三方库,例如:
```
npm install vue-router --save
npm install axios --save
npm install echarts --save
```
3. 在 src 目录下,创建一个名为 components 的新目录,用于存放 Vue 组件。
4. 在 components 目录下,创建一个名为 Report.vue 的新文件,用于编写商品报表页面的 Vue 组件。
5. 在 Report.vue 文件中,添加必要的代码,例如:
```
<template>
<div>
<h1>销售报表</h1>
<table>
<thead>
<tr>
<th>商品名称</th>
<th>销售数量</th>
<th>销售额</th>
</tr>
</thead>
<tbody>
<tr v-for="item in data" :key="item.id">
<td>{{ item.name }}</td>
<td>{{ item.quantity }}</td>
<td>{{ item.amount }}</td>
</tr>
</tbody>
</table>
<div ref="chart" style="height: 400px;"></div>
<p>销售额单位:万元</p>
</div>
</template>
<script>
import axios from 'axios'
import echarts from 'echarts'
export default {
data() {
return {
data: []
}
},
async created() {
const response = await axios.get('/api/report')
this.data = response.data
this.renderChart()
},
methods: {
renderChart() {
const chart = echarts.init(this.$refs.chart)
const option = {
title: {
text: '销售额'
},
tooltip: {},
xAxis: {
data: this.data.map(item => item.name)
},
yAxis: {},
series: [{
name: '销售额',
type: 'bar',
data: this.data.map(item => item.amount)
}]
}
chart.setOption(option)
}
}
}
</script>
```
6. 在 src 目录下,创建一个名为 router 的新目录,用于存放 Vue Router 相关代码。
7. 在 router 目录下,创建一个名为 index.js 的新文件,用于配置路由。
```
import Vue from 'vue'
import VueRouter from 'vue-router'
import Report from '@/components/Report.vue'
Vue.use(VueRouter)
const routes = [
{
path: '/',
name: 'report',
component: Report
}
]
const router = new VueRouter({
mode: 'history',
routes
})
export default router
```
8. 在 src 目录下,创建一个名为 App.vue 的新文件,用于组合和渲染 Vue 组件。
```
<template>
<div id="app">
<router-view></router-view>
</div>
</template>
<script>
export default {
name: 'app'
}
</script>
```
9. 在 main.js 文件中,引入 Vue、Vue Router 和 App 组件,并创建一个新的 Vue 实例。
```
import Vue from 'vue'
import App from './App.vue'
import router from './router'
Vue.config.productionTip = false
new Vue({
router,
render: h => h(App)
}).$mount('#app')
```
10. 在后端服务器中,提供一个名为 /api/report 的 API 接口,用于返回商品报表的数据,例如:
```
const data = [
{ id: 1, name: '商品A', quantity: 100, amount: 10000 },
{ id: 2, name: '商品B', quantity: 200, amount: 20000 }
]
app.get('/api/report', (req, res) => {
res.json(data)
})
```
以上就是使用 Vue 制作商品报表页面的基本步骤,您可以根据实际需求进行修改和完善。
阅读全文
相关推荐
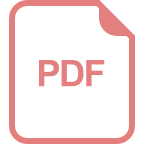
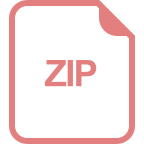
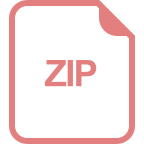
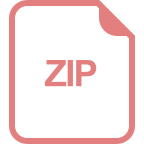
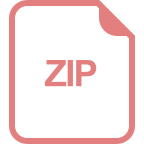
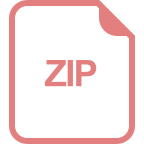
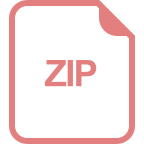
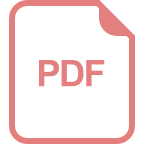
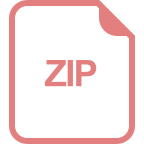
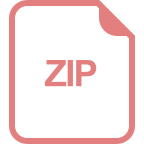
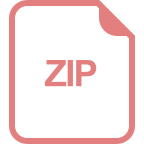
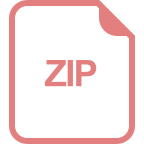
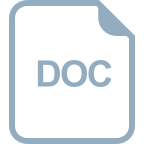
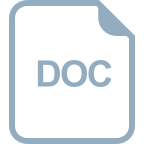
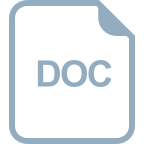
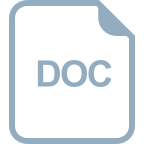
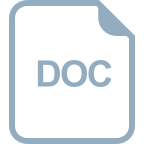
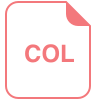