jquery淡入淡出轮播图jquery DOM操作
时间: 2023-08-01 08:11:14 浏览: 110
好的,下面是一个 jQuery DOM 操作的代码示例,使用 jQuery 动态生成轮播图的 HTML 结构:
HTML 代码:
```html
<div class="slider">
<div class="slides"></div>
<div class="dots"></div>
</div>
```
CSS 代码:
```css
.slider {
position: relative;
height: 500px;
}
.slides {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
}
.slides img {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
opacity: 0;
transition: opacity 1s ease;
}
.slides img.active {
opacity: 1;
}
.dots {
position: absolute;
bottom: 20px;
left: 50%;
transform: translateX(-50%);
}
.dot {
display: inline-block;
width: 10px;
height: 10px;
border-radius: 50%;
background-color: #fff;
margin-right: 10px;
opacity: 0.5;
transition: opacity 0.5s ease;
}
.dot.active {
opacity: 1;
}
```
jQuery 代码:
```javascript
$(function() {
var slider = $('.slider');
var slides = slider.find('.slides');
var dots = slider.find('.dots');
var slideCount = 3;
var currentIndex = 0;
// 动态生成轮播图的 HTML 结构
for (var i = 1; i <= slideCount; i++) {
var slide = $('<img>').attr('src', 'image' + i + '.jpg').attr('alt', 'Image ' + i);
slides.append(slide);
var dot = $('<span>').addClass('dot').data('index', i - 1);
dots.append(dot);
}
slides.find('img:first-child').addClass('active');
dots.find('.dot:first-child').addClass('active');
// 点击小圆点切换图片
dots.on('click', '.dot', function() {
var index = $(this).data('index');
currentIndex = index;
showSlide(currentIndex);
});
function showSlide(index) {
slides.find('img').removeClass('active');
slides.find('img').eq(index).addClass('active');
dots.find('.dot').removeClass('active');
dots.find('.dot').eq(index).addClass('active');
}
function nextSlide() {
currentIndex = (currentIndex + 1) % slideCount;
showSlide(currentIndex);
}
setInterval(nextSlide, 3000);
});
```
这段代码会动态生成轮播图的 HTML 结构,包括图片和小圆点,并且可以点击小圆点切换图片。你可以替换 HTML 代码中的图片链接来展示自己的图片。
阅读全文
相关推荐
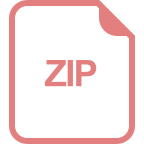
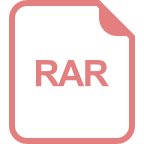
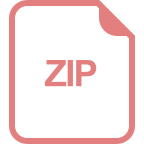
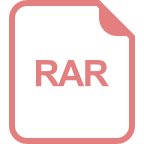
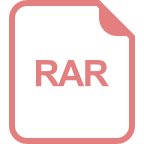
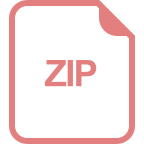
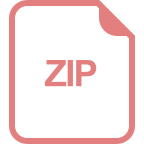
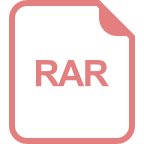
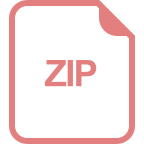
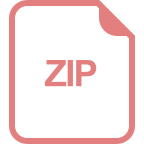
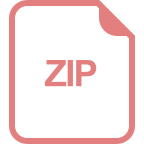
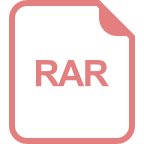
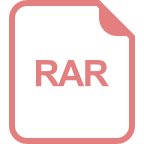
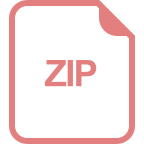
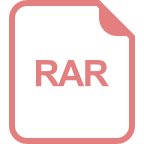
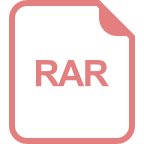