从键盘输入年份和月份,输出该月份的日历表
时间: 2024-05-15 20:12:15 浏览: 9
好的,我可以帮你实现这个功能。以下是一个 Python 代码示例:
```python
import calendar
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
cal = calendar.monthcalendar(year, month)
print("{0}年{1}月的日历表:".format(year, month))
print("Mo Tu We Th Fr Sa Su")
for week in cal:
line = ""
for day in week:
if day == 0:
line += " "
else:
line += "{:2d} ".format(day)
print(line)
```
运行程序后,你需要输入年份和月份。程序将使用 Python 的 `calendar` 模块生成该月份的日历表,并输出到屏幕上。
注意,这个程序只支持公历。如果你需要支持其他历法,你需要使用相应的库或算法来计算日期。
相关问题
从键盘输入年份和月份, 输出该月份的日历表
好的,我可以为您提供一个Python代码实现打印月历的功能。以下是代码:
```python
import calendar
# 输入年份和月份
year = int(input("请输入年份: "))
month = int(input("请输入月份: "))
# 打印该月份的日历表
print(calendar.month(year, month))
```
您可以在命令行或控制台中运行此代码,并根据提示输入要查看的年份和月份。程序将使用`calendar`模块中的`month()`函数打印出该月份的完整日历表。
java从键盘输入年份和月份, 输出该月份的日历表:
实现该功能的一种方法是使用Java中的Calendar类和SimpleDateFormat类。以下是一个示例代码,它可以从键盘输入年份和月份,并打印出该月份的日历表:
```java
import java.util.Calendar;
import java.util.Scanner;
public class CalendarApp {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入年份: ");
int year = scanner.nextInt();
System.out.print("请输入月份: ");
int month = scanner.nextInt();
// 创建一个Calendar对象,设置日期为当月的第一天
Calendar calendar = Calendar.getInstance();
calendar.set(year, month - 1, 1);
// 获取当月的天数
int daysInMonth = calendar.getActualMaximum(Calendar.DAY_OF_MONTH);
// 获取当月第一天是星期几
int firstDayOfWeek = calendar.get(Calendar.DAY_OF_WEEK);
// 打印日历表头
System.out.println("日\t一\t二\t三\t四\t五\t六");
// 打印日历表格
int dayOfMonth = 1;
for (int i = 1; i <= 6; i++) {
for (int j = 1; j <= 7; j++) {
if (i == 1 && j < firstDayOfWeek) {
System.out.print("\t");
} else if (dayOfMonth > daysInMonth) {
break;
} else {
System.out.print(dayOfMonth + "\t");
dayOfMonth++;
}
}
System.out.println();
}
}
}
```
运行程序后,它会要求你输入年份和月份。输入后,程序会输出该月份的日历表。例如,输入2022和3,程序将输出以下内容:
```
请输入年份: 2022
请输入月份: 3
日 一 二 三 四 五 六
1 2 3 4 5
6 7 8 9 10 11 12
13 14 15 16 17 18 19
20 21 22 23 24 25 26
27 28 29 30 31
```
注意,该代码只是一种实现方式,还有其他方法可以实现该功能。
相关推荐
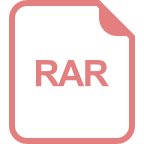






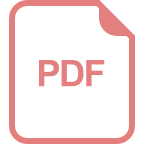
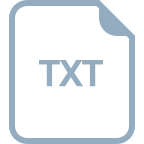
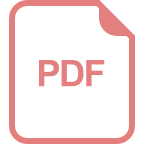