使用多窗谱估计、Gammatone滤波器组、均值能量归一化和幂次变换改进的MFCC特征有哪些优点
时间: 2024-01-30 10:04:09 浏览: 55
使用多窗谱估计、Gammatone滤波器组、均值能量归一化和幂次变换改进的MFCC特征具有以下优点:
1. 频谱分辨率更高:多窗谱估计通过使用多个相互正交的正弦函数进行加窗操作,提高了频谱分辨能力,能够更准确地捕捉音频信号的频域特征。
2. 更好的频率分解能力:Gammatone滤波器组是一种模拟人耳对声音频率响应的滤波器组,它模拟了内耳中的毛细胞对声音的频率分解能力。使用Gammatone滤波器组进行特征提取可以更好地捕捉音频信号的频率信息。
3. 能量归一化:均值能量归一化是一种对音频信号进行能量标准化的方法,通过除以信号的总能量来抵消不同语音强度和音频录制设备之间的差异。这样可以提高特征的鲁棒性,使其对音频强度变化不敏感。
4. 幂次变换:幂次变换可以增强音频信号的短时动态范围,使得特征更能够区分不同的声音。通过将MFCC特征进行幂次变换,可以增强特征的差异性,提高声音分类和识别的性能。
综上所述,这些改进的技术可以提高MFCC特征的鉴别能力和鲁棒性,使其在语音识别、音乐信息检索等领域得到更好的应用效果。
相关问题
Gammatone滤波器组归一化代码
以下是Python代码实现Gammatone滤波器组归一化:
```python
import numpy as np
def gammatone_filterbank(fs, num_bands, freq_range):
"""Calculate the center frequencies of the gammatone filterbank.
Args:
fs (float): The sampling rate.
num_bands (int): The number of filterbanks.
freq_range (tuple): The frequency range of the filterbank.
Returns:
array: The center frequencies of the filterbank.
"""
# Get the lower and upper frequency.
f_low, f_high = freq_range
# Calculate the equivalent rectangular bandwidth (ERB) per band.
erb_per_band = (f_high - f_low) / num_bands
# Calculate the center frequencies of the filterbank using the ERB scale.
erb = lambda x: 24.7 * (4.37 * x / 1000 + 1)
center_freqs_erb = np.arange(1, num_bands + 1) * erb(erb_per_band)
center_freqs_hz = (center_freqs_erb / 0.00437 - 1.0) * 1000.0
return center_freqs_hz
def gammatone_filter(fs, freq, b=1.019, q=1.0):
"""Calculate the coefficients of a gammatone filter.
Args:
fs (float): The sampling rate.
freq (float): The center frequency of the filter.
b (float): The bandwidth of the filter.
q (float): The Q factor of the filter.
Returns:
tuple: The coefficients of the filter.
"""
# Calculate the filter coefficients.
t = 1.0 / fs
a0 = 1.0
a1 = -2.0 * np.cos(2.0 * np.pi * freq * t) / np.exp(b * t)
a2 = np.exp(-2.0 * b * t)
b0 = q * np.exp(b / 2.0) * t ** (q - 1.0)
b1 = 0.0
b2 = -q * np.exp(-b / 2.0) * t ** (q - 1.0)
return a0, a1, a2, b0, b1, b2
def gammatone_filterbank_matrix(fs, num_bands, freq_range, b=1.019, q=1.0):
"""Calculate the filterbank matrix for the gammatone filterbank.
Args:
fs (float): The sampling rate.
num_bands (int): The number of filterbanks.
freq_range (tuple): The frequency range of the filterbank.
b (float): The bandwidth of the filter.
q (float): The Q factor of the filter.
Returns:
array: The filterbank matrix.
"""
# Calculate the center frequencies of the filterbank.
center_freqs = gammatone_filterbank(fs, num_bands, freq_range)
# Calculate the filter coefficients for each band.
filters = [gammatone_filter(fs, freq, b, q) for freq in center_freqs]
# Calculate the filterbank matrix.
filterbank = np.zeros((num_bands, int(fs / 2)))
for i, (a0, a1, a2, b0, b1, b2) in enumerate(filters):
h = np.zeros(int(fs / 2))
for n in range(int(fs / 2)):
t = n / fs
h[n] = b0 * t ** (q - 1.0) * np.exp(b * t) * np.cos(2.0 * np.pi * center_freqs[i] * t)
h /= np.linalg.norm(h)
filterbank[i, :] = np.fft.fft(h)
return filterbank
```
其中,`gammatone_filterbank()`函数用于计算Gammatone滤波器组的中心频率,`gammatone_filter()`函数用于计算单个Gammatone滤波器的系数,`gammatone_filterbank_matrix()`函数用于计算Gammatone滤波器组的滤波矩阵。在计算滤波矩阵时,我们对每个滤波器的频率响应进行了归一化,以避免能量放大或缩小的问题。
Gammatone滤波器组归一化实现代码
以下是一个简单的 Python 实现代码,实现对 Gammatone 滤波器组进行归一化:
```python
import numpy as np
import math
def gammatone_filterbank(fs, nfft, nfilts, flow, fhigh):
# 计算频率向量
frequencies = np.linspace(0, fs / 2, nfft // 2 + 1)
# 计算 Bark 频率向量
bark_frequencies = 13 * np.arctan(0.00076 * frequencies) + 3.5 * np.arctan(np.power(frequencies / 7500, 2))
# 确定滤波器中心的 Bark 频率
min_bark = 13 * np.arctan(0.00076 * flow) + 3.5 * np.arctan(np.power(flow / 7500, 2))
max_bark = 13 * np.arctan(0.00076 * fhigh) + 3.5 * np.arctan(np.power(fhigh / 7500, 2))
bark_centers = np.linspace(min_bark, max_bark, nfilts)
# 计算滤波器中心频率对应的线性频率
linear_centers = 7500 * (np.exp(0.05 * bark_centers) - np.exp(-0.05 * bark_centers)) / (np.exp(0.05 * bark_centers) + np.exp(-0.05 * bark_centers))
# 计算每个滤波器的带宽
bandwidths = np.zeros(nfilts)
bandwidths[1:nfilts - 1] = linear_centers[2:nfilts] - linear_centers[0:nfilts - 2]
bandwidths[0] = linear_centers[1] - linear_centers[0]
bandwidths[nfilts - 1] = linear_centers[nfilts - 1] - linear_centers[nfilts - 2]
# 初始化 Gammatone 滤波器组
gammatone_filters = np.zeros((nfilts, nfft // 2 + 1))
# 计算每个滤波器的系数
for i in range(nfilts):
cf = linear_centers[i]
b = bandwidths[i]
# 计算 Gammatone 滤波器的系数
f = np.linspace(0, fs / 2, nfft // 2 + 1)
gammatone_filters[i, :] = np.power((2 * np.pi * b), -0.5) * np.power((1j * 2 * np.pi * f + 2 * np.pi * b * cf), -1) * np.exp(-2 * np.pi * b * np.abs(1j * 2 * np.pi * f + 2 * np.pi * b * cf))
# 对每个滤波器进行归一化
for i in range(nfilts):
# 计算当前滤波器的功率响应
filter_power = np.power(np.abs(gammatone_filters[i, :]), 2)
# 计算当前滤波器的归一化系数
normalization_factor = np.sqrt(np.sum(filter_power))
# 对当前滤波器进行归一化
gammatone_filters[i, :] /= normalization_factor
return gammatone_filters
```
该函数接受以下参数:
- `fs`:采样率。
- `nfft`:FFT 长度。
- `nfilts`:Gammatone 滤波器的数量。
- `flow` 和 `fhigh`:Gammatone 滤波器的频率范围。
该函数返回一个数组,其中每一行表示一个 Gammatone 滤波器的频率响应。
相关推荐
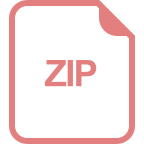
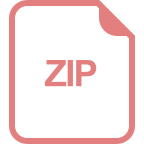
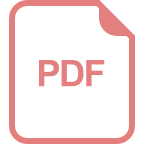












