c语言编写单词搜索软件
时间: 2023-08-11 15:04:13 浏览: 83
好的,编写单词搜索软件可以分为以下几个步骤:
1. 读取文本文件中的单词和字符矩阵
可以使用C标准库中的文件读取函数,如fopen、fread等函数读取文件内容,将单词和字符矩阵分别存储到数组中。
2. 实现单词搜索功能
可以采用暴力搜索的方式,遍历矩阵中的每个字符,以该字符为起点,依次向其上、下、左、右、左上、左下、右上和右下八个方向搜索,直到找到单词或者搜索到矩阵的边界。
3. 输出搜索结果
将搜索得到的单词输出到文件或者控制台上。
下面是一个简单的示例代码,用于实现单词搜索功能:
```c
#include <stdio.h>
#include <string.h>
#define MAX_WORD_LEN 20
#define MAX_ROWS 10
#define MAX_COLS 10
char words[100][MAX_WORD_LEN + 1];
char matrix[MAX_ROWS][MAX_COLS];
void search_word(int r, int c, int row, int col, int len, char *word)
{
if (len == strlen(word)) {
printf("Found word: %s\n", word);
return;
}
if (r < 0 || r >= row || c < 0 || c >= col)
return;
if (matrix[r][c] != word[len])
return;
char temp = matrix[r][c];
matrix[r][c] = ' ';
search_word(r - 1, c, row, col, len + 1, word);
search_word(r + 1, c, row, col, len + 1, word);
search_word(r, c - 1, row, col, len + 1, word);
search_word(r, c + 1, row, col, len + 1, word);
search_word(r - 1, c - 1, row, col, len + 1, word);
search_word(r - 1, c + 1, row, col, len + 1, word);
search_word(r + 1, c - 1, row, col, len + 1, word);
search_word(r + 1, c + 1, row, col, len + 1, word);
matrix[r][c] = temp;
}
int main()
{
int n, row, col;
printf("Enter the number of words: ");
scanf("%d", &n);
printf("Enter the words:\n");
for (int i = 0; i < n; i++)
scanf("%s", words[i]);
printf("Enter the number of rows: ");
scanf("%d", &row);
printf("Enter the number of cols: ");
scanf("%d", &col);
printf("Enter the matrix:\n");
for (int i = 0; i < row; i++)
scanf("%s", matrix[i]);
for (int i = 0; i < n; i++) {
for (int j = 0; j < row; j++) {
for (int k = 0; k < col; k++) {
if (matrix[j][k] == words[i][0])
search_word(j, k, row, col, 0, words[i]);
}
}
}
return 0;
}
```
注意:以上仅为示例代码,还有很多可以优化的地方,比如可以使用Trie树来存储单词,使用更高效的搜索算法等。
相关推荐
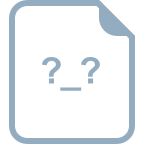
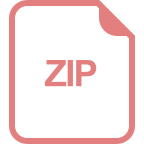














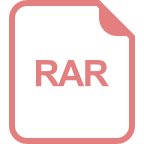