public static void copyFilesFromAssets(Context context, String filePath) { File jhPath = new File(filePath); //查看该文件是否存在 if (jhPath.exists()) { Log.e("test", "该文件已存在"); } else { Log.e("test", "该文件不存在"); try { //得到资源 AssetManager am = context.getAssets(); //得到该文件的输入流 InputStream is = am.open("help.pdf"); Log.e("test", is + ""); //用输出流写到特定路径下 FileOutputStream fos = new FileOutputStream(jhPath); // Log.e("test", "fos="+fos); // Log.e("test", "jhPath="+jhPath); //创建byte数组 用于1KB写一次 byte[] buffer = new byte[1024]; int count = 0; while ((count = is.read(buffer)) > 0) { fos.write(buffer, 0, count); } //最后关闭流 fos.flush(); fos.close(); is.close(); } catch (IOException e) { e.printStackTrace(); } } }优化
时间: 2024-02-10 09:15:39 浏览: 28
这段代码的功能是从Assets目录下复制一个文件到指定路径。以下是对该代码的一些优化建议:
1. 将方法名改为更具体的名称,例如copyFileFromAssetsToPath,以更好地表达该方法的作用。
2. 将“help.pdf”硬编码的字符串改为参数传入,使该方法更加通用。
3. 使用try-with-resources语句自动关闭流,避免手动关闭流时可能出现的异常。
4. 在while循环中使用缓冲区的实际读取长度作为写入长度,避免可能出现的空字节写入。
优化后的代码如下:
```
public static void copyFileFromAssetsToPath(Context context, String fileName, String filePath) {
File destFile = new File(filePath);
if (destFile.exists()) {
Log.e("test", "The file already exists");
} else {
try (InputStream is = context.getAssets().open(fileName);
FileOutputStream fos = new FileOutputStream(destFile)) {
byte[] buffer = new byte[1024];
int length;
while ((length = is.read(buffer)) != -1) {
fos.write(buffer, 0, length);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
相关推荐
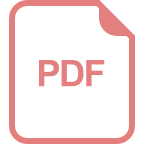
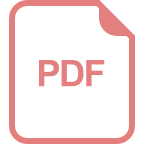
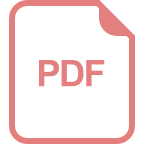















