jquery结合数据库实现简单的留言板
时间: 2024-01-24 13:04:26 浏览: 73
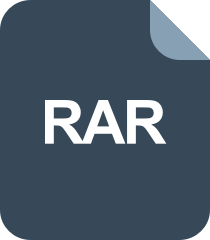
一款较简单的留言板系统带数据库
好的,下面是一个简单的留言板实现示例,使用jQuery和PHP结合MySQL数据库实现:
HTML代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>留言板</title>
<meta charset="UTF-8">
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script src="js/message_board.js"></script>
</head>
<body>
<h1>留言板</h1>
<form id="message_form">
<label for="name">姓名:</label>
<input type="text" id="name" name="name"><br><br>
<label for="content">留言:</label>
<textarea id="content" name="content"></textarea><br><br>
<input type="button" id="submit_btn" value="提交">
</form>
<div id="message_list">
<!-- 留言列表将在此处显示 -->
</div>
</body>
</html>
```
JavaScript代码(message_board.js):
```javascript
$(function() {
// 页面加载完成后,获取留言列表
getMessages();
// 点击提交按钮,提交留言
$("#submit_btn").click(function() {
var name = $("#name").val();
var content = $("#content").val();
if (name.trim() == "" || content.trim() == "") {
alert("姓名和留言内容不能为空!");
return;
}
$.post("php/add_message.php", {name: name, content: content}, function(data) {
if (data == "success") {
alert("留言提交成功!");
$("#name").val("");
$("#content").val("");
getMessages();
} else {
alert("留言提交失败!");
}
});
});
});
// 获取留言列表
function getMessages() {
$.get("php/get_messages.php", function(data) {
$("#message_list").html(data);
});
}
```
PHP代码(add_message.php):
```php
<?php
// 连接数据库
$servername = "localhost";
$username = "root";
$password = "123456";
$dbname = "message_board";
$conn = mysqli_connect($servername, $username, $password, $dbname);
if (!$conn) {
die("连接失败: " . mysqli_connect_error());
}
// 获取POST数据
$name = $_POST["name"];
$content = $_POST["content"];
// 插入数据
$sql = "INSERT INTO messages (name, content) VALUES ('$name', '$content')";
if (mysqli_query($conn, $sql)) {
echo "success";
} else {
echo "error: " . $sql . "<br>" . mysqli_error($conn);
}
// 关闭连接
mysqli_close($conn);
?>
```
PHP代码(get_messages.php):
```php
<?php
// 连接数据库
$servername = "localhost";
$username = "root";
$password = "123456";
$dbname = "message_board";
$conn = mysqli_connect($servername, $username, $password, $dbname);
if (!$conn) {
die("连接失败: " . mysqli_connect_error());
}
// 获取留言列表
$sql = "SELECT * FROM messages ORDER BY id DESC";
$result = mysqli_query($conn, $sql);
if (mysqli_num_rows($result) > 0) {
// 输出留言列表
while($row = mysqli_fetch_assoc($result)) {
echo "<div>";
echo "<p>" . $row["name"] . "</p>";
echo "<p>" . $row["content"] . "</p>";
echo "<p>" . $row["create_time"] . "</p>";
echo "</div>";
}
} else {
echo "暂无留言!";
}
// 关闭连接
mysqli_close($conn);
?>
```
这个留言板实现比较简单,只有基本的增加留言和显示留言列表功能,如果需要更多的功能,可以根据需要进行扩展。
阅读全文
相关推荐
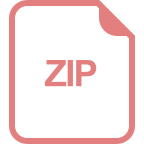
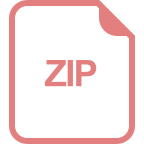
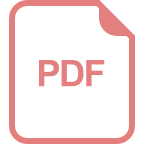
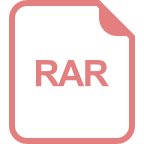
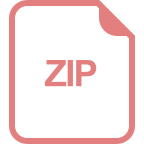
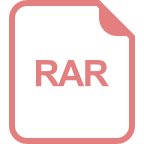
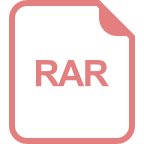
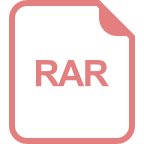
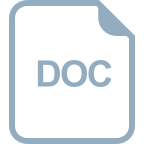
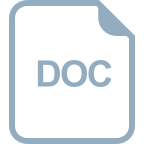
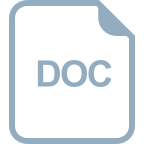
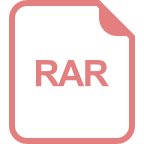
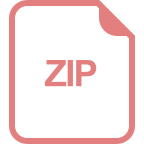
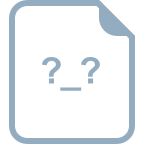
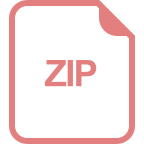
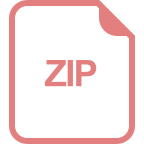
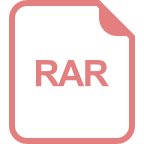