import tkinter as tk from tkinter import filedialog from PIL import ImageTk, Image # 创建窗口 window = tk.Tk() window.title("宝石预测") window.geometry("400x400") # 加载模型参数 para_state_dict = paddle.load("MyCNN") model = MyCNN() model.set_state_dict(para_state_dict) model.eval() # 加载标签字典 label_dict = train_parameters['label_dict'] # 创建预测函数 def predict(): # 获取待预测图片路径 img_path = filedialog.askopenfilename() img = Image.open(img_path) # 预处理图片 img = img.resize((224, 224), Image.BILINEAR) img = np.array(img).astype('float32') img = img.transpose((2, 0, 1)) img = img / 255 img = np.array([img]) # 进行预测 img = paddle.to_tensor(img) out = model(img) label = np.argmax(out.numpy()) result = label_dict[str(label)] # 显示预测结果 result_label.config(text="预测结果:{}".format(result)) # 显示待预测图片 img = ImageTk.PhotoImage(Image.open(img_path).resize((200, 200))) img_label.config(image=img) img_label.image = img # 创建选择图片按钮 select_button = tk.Button(window, text="选择图片", command=predict) select_button.pack(pady=20) # 创建待预测图片区域 img_label = tk.Label(window) img_label.pack() # 创建预测结果区域 result_label = tk.Label(window, font=("Helvetica", 16)) result_label.pack(pady=20) # 进入消息循环 window.mainloop()在此基础上添加预测结果与实际结果对比判断是否预测正确的功能
时间: 2023-12-09 15:06:11 浏览: 70
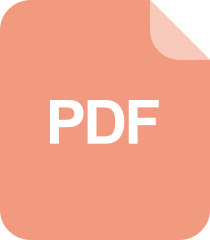
Python3.4 tkinter,PIL图片转换
可以在预测函数中添加对比代码,如下所示:
```python
def predict():
# 获取待预测图片路径
img_path = filedialog.askopenfilename()
img = Image.open(img_path)
# 预处理图片
img = img.resize((224, 224), Image.BILINEAR)
img = np.array(img).astype('float32')
img = img.transpose((2, 0, 1))
img = img / 255
img = np.array([img])
# 进行预测
img = paddle.to_tensor(img)
out = model(img)
label = np.argmax(out.numpy())
result = label_dict[str(label)]
# 对比预测结果和实际结果
actual_label = label_dict[img_path.split('/')[-2]]
if result == actual_label:
compare_result = "预测正确"
else:
compare_result = "预测错误"
# 显示预测结果和对比结果
result_label.config(text="预测结果:{},{}".format(result, compare_result))
# 显示待预测图片
img = ImageTk.PhotoImage(Image.open(img_path).resize((200, 200)))
img_label.config(image=img)
img_label.image = img
```
其中,`actual_label` 表示待预测图片的实际标签,通过图片路径获取。`compare_result` 表示预测结果和实际结果的对比结果,如果相同则为“预测正确”,否则为“预测错误”。在显示预测结果时,将对比结果一并显示出来。
阅读全文
相关推荐
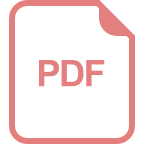
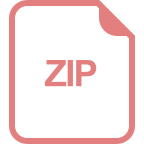














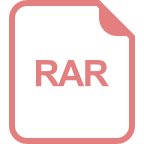