编写修改用户名,密码功能,提示用户输入旧的用户名和密码,如果正确,提示输入新的用户名和密码,并通过输入输出流更新存在d盘save.txt文件中的用户名和密码
时间: 2024-02-25 11:57:19 浏览: 39
好的,这个功能需要用到Java的文件读写和字符串操作,下面是一个简单的实现:
```java
import java.io.*;
public class ModifyUser {
public static void main(String[] args) {
// 从控制台读入旧的用户名和密码
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
System.out.print("请输入旧的用户名:");
String oldUsername = null;
try {
oldUsername = reader.readLine();
} catch (IOException e) {
e.printStackTrace();
}
System.out.print("请输入旧的密码:");
String oldPassword = null;
try {
oldPassword = reader.readLine();
} catch (IOException e) {
e.printStackTrace();
}
// 读取文件中的用户名和密码
String filePath = "D:\\save.txt";
String[] userPass = readUserPass(filePath);
String username = userPass[0];
String password = userPass[1];
// 检查旧的用户名和密码是否匹配
if (!username.equals(oldUsername) || !password.equals(oldPassword)) {
System.out.println("用户名或密码错误!");
return;
}
// 提示用户输入新的用户名和密码
System.out.print("请输入新的用户名:");
String newUsername = null;
try {
newUsername = reader.readLine();
} catch (IOException e) {
e.printStackTrace();
}
System.out.print("请输入新的密码:");
String newPassword = null;
try {
newPassword = reader.readLine();
} catch (IOException e) {
e.printStackTrace();
}
// 把新的用户名和密码写入文件
writeUserPass(filePath, newUsername, newPassword);
System.out.println("用户名和密码修改成功!");
}
private static String[] readUserPass(String filePath) {
String[] userPass = new String[2];
try {
BufferedReader reader = new BufferedReader(new FileReader(filePath));
userPass[0] = reader.readLine();
userPass[1] = reader.readLine();
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
return userPass;
}
private static void writeUserPass(String filePath, String username, String password) {
try {
BufferedWriter writer = new BufferedWriter(new FileWriter(filePath));
writer.write(username);
writer.newLine();
writer.write(password);
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在这个实现中,我们首先从控制台读入旧的用户名和密码,并通过``readUserPass()``函数读取文件中保存的用户名和密码。然后我们检查旧的用户名和密码是否匹配,如果不匹配则直接提示错误。接下来,我们通过``writeUserPass()``函数把新的用户名和密码写入文件。注意这里使用了``BufferedReader``和``BufferedWriter``来进行文件读写,这样可以提高读写效率。
这个实现中还有很多可以优化的地方,比如错误处理、输入校验等等,但是这应该可以满足你的需求了。
相关推荐
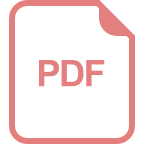
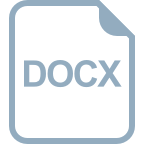
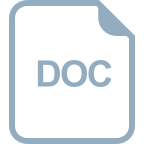














