#include <iostream> using namespace std; class Point { private: int x; // 横坐标 int y; // 纵坐标 public: Point() { x = 0; y = 0; } Point(int xx, int yy) { x = xx; y = yy; } Point(const Point& p) { x = p.x; y = p.y; } void offert(int dx, int dy) { x += dx; y += dy; } void offert(Point& p) { x += p.x; y += p.y; } bool operator==(Point& p) { return x == p.x && y == p.y; } bool operator!=(Point& p) { return !(*this == p); } void operator+=(Point& p) { x += p.x; y += p.y; } void operator-=(Point& p) { x -= p.x; y -= p.y; } void operator++() { x++; y++; } void operator--() { x--; y--; } Point operator++(int k) { Point tmp(*this); x++; y++; return tmp; } Point operator--(int k) { Point tmp(*this); x--; y--; return tmp; } friend Point& operator+(Point& p1, Point& p2) { Point tmp(p1.x + p2.x, p1.y + p2.y); return tmp; } friend Point& operator-(Point& p1, Point& p2) { Point tmp(p1.x - p2.x, p1.y - p2.y); return tmp; } int GetX() { return x; } int GetY() { return y; } void Display() { cout << "(" << x << ", " << y << ")" << endl; } };
时间: 2024-02-14 15:22:34 浏览: 170
这是一个 C++ 的程序,定义了一个 Point 类,表示一个二维平面上的点。这个类有两个私有成员变量 x 和 y 分别表示横坐标和纵坐标,以及多个成员函数和运算符重载函数,如构造函数、坐标偏移函数、相等判断运算符、不等判断运算符、加法运算符、减法运算符等等。此外,这个类还有一些友元函数,用来实现 Point 类对象的加法、减法运算。
相关问题
分析以下代码#include <iostream> #include <memory> using namespace std; class Point { public: Point(int ix = 0, int iy = 0): _ix(ix), _iy(iy) { cout << "Point(int,int)" << endl; } shared_ptr<Point> addPoint(Point & pt) { _ix += pt._ix; _iy += pt._iy; return shared_ptr<Point>(this); } ~Point() { cout << "~Point()" << endl; } private: int _ix; int _iy; }; int main() { shared_ptr<Point> sp(new Point(1, 2)); shared_ptr<Point> sp2(new Point(11, 12)); shared_ptr<Point> sp3(sp->addPoint(*sp2)); }
这段代码定义了一个 Point 类,其中包含两个私有变量 _ix 和 _iy 分别表示点的横坐标和纵坐标。在 main 函数中,分别定义了三个 shared_ptr 智能指针对象 sp、sp2 和 sp3,其中 sp 和 sp2 分别指向两个 Point 类型的对象,分别是 (1, 2) 和 (11, 12)。然后通过调用 sp 的成员函数 addPoint 传入 sp2 对象,并将结果保存在 sp3 中,addPoint 函数实现了将两个点的坐标相加的功能,并返回指向 this 的 shared_ptr 智能指针对象。
需要注意的是,addPoint 函数返回指向 this 的 shared_ptr 智能指针对象,这意味着 Point 类对象的生命周期将由 sp3 管理,因此需要确保在 addPoint 函数返回后,sp 和 sp2 指向的对象不再被使用,否则会出现悬空指针问题。同时,由于 sp3 持有了两个 Point 类型对象的所有权,因此需要使用 shared_ptr 来管理对象的生命周期,以避免内存泄漏。
#include < iostream > using namespace std; class Point { private: //访问权限设置,私有权限 int x;//横坐标 int y;//纵坐标 public: //访问权限设置,公有权限 //以下为构造函数,用参数a,b分别为横纵坐标进行初始化 Point (int a,int b) { x=a ; y=b ; } //以下为拷贝构造函数,借用对象a_point完成初始化 Point( const Point & a_point) { x=a_point.x; y=a_point.y; } //以下为析构函数 ~Point { cout<<"Deconstructed Point"; print(); } //以下为输出点的信息的函数,要求在一行中输出点的坐标信息,形如:(横坐标,纵坐标) void print() { cout<< x <<","<< ycon <<endl; } }; int main() { Point b_point(0,0); b_point.print(); int a,b; //从标准输入流中提取数值给a,b Point c_point(a,b); c_point.print(); //主函数的返回语句 } /*设输入为10 10,则本程序的运行结果为: */完善这段代码
这段代码存在一些问题,需要进行完善:
1. 在main函数中,没有从标准输入流中读入a和b的值就直接使用了,需要在读入a和b的语句前加上如下代码:
```cpp
cin >> a >> b;
```
2. 在Point类的析构函数中,需要在类名后面添加一对小括号,即:
```cpp
~Point()
```
3. 在print函数中,输出语句中的变量名错误,应该是y而不是ycon,应该修改为:
```cpp
cout << x << "," << y << endl;
```
修改后的代码如下所示:
```cpp
#include <iostream>
using namespace std;
class Point {
private:
int x; //横坐标
int y; //纵坐标
public:
//以下为构造函数,用参数a,b分别为横纵坐标进行初始化
Point(int a, int b)
{
x = a;
y = b;
}
//以下为拷贝构造函数,借用对象a_point完成初始化
Point(const Point& a_point)
{
x = a_point.x;
y = a_point.y;
}
//以下为析构函数
~Point()
{
cout << "Deconstructed Point";
print();
}
//以下为输出点的信息的函数,要求在一行中输出点的坐标信息,形如:(横坐标,纵坐标)
void print()
{
cout << x << "," << y << endl;
}
};
int main()
{
Point b_point(0, 0);
b_point.print();
int a, b;
cin >> a >> b;
Point c_point(a, b);
c_point.print();
return 0; //主函数的返回语句
}
```
阅读全文
相关推荐
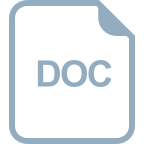
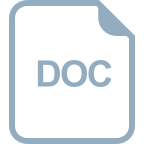
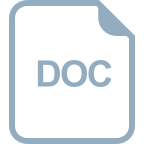













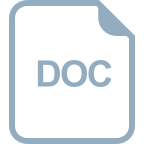