如何用springboot 和RabbitMQ向指定用户发送通知,前端作为消费者。可以给示例代码吗,包括交换机的配置,后端如何生产消息和前端如何消费消息
时间: 2023-08-31 07:24:19 浏览: 73
### 回答1:
Spring Boot 集成 RabbitMQ 的过程可以分为三个步骤:
1. 在 pom.xml 文件中添加 RabbitMQ 的依赖。
2. 配置 RabbitMQ 连接工厂,包括交换机,队列和路由键。
3. 编写生产者代码和消费者代码。
以下是示例代码:
1. pom.xml 文件中添加 RabbitMQ 的依赖
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-amqp</artifactId>
</dependency>
```
2. 配置 RabbitMQ 连接工厂
```java
@Configuration
public class RabbitMQConfig {
@Value("${rabbitmq.queue}")
private String queueName;
@Value("${rabbitmq.exchange}")
private String exchange;
@Value("${rabbitmq.routingkey}")
private String routingkey;
@Bean
Queue queue() {
return new Queue(queueName, false);
}
@Bean
DirectExchange exchange() {
return new DirectExchange(exchange);
}
@Bean
Binding binding(Queue queue, DirectExchange exchange) {
return BindingBuilder.bind(queue).to(exchange).with(routingkey);
}
}
```
3. 编写生产者代码
```java
@Service
public class RabbitMQSender {
@Autowired
private AmqpTemplate rabbitTemplate;
@Value("${rabbitmq.exchange}")
private String exchange;
@Value("${rabbitmq.routingkey}")
private String routingkey;
public void send(User user) {
rabbitTemplate.convertAndSend(exchange, routingkey, user);
}
}
```
4. 编写消费者代码
```java
@Service
public class RabbitMQConsumer {
@RabbitListener(queues = "${rabbitmq.queue}")
public void recievedMessage(User user) {
// 根据 user 中的信息发送通知
}
}
```
在前端消费者可以使用 WebSocket,与后端建立连接,实时监听消息,并展示给用户。
注
### 回答2:
在使用Spring Boot和RabbitMQ向指定用户发送通知的情况下,需要配置交换机、生产消息的后端和消费消息的前端。
1. 配置交换机:
```java
@Configuration
public class RabbitMQConfig {
@Bean
public DirectExchange directExchange() {
return new DirectExchange("direct_exchange");
}
}
```
2. 后端生产消息:
```java
@Service
public class NotificationService {
@Autowired
private RabbitTemplate rabbitTemplate;
public void sendNotification(String userId, String message) {
rabbitTemplate.convertAndSend("direct_exchange", userId, message);
}
}
```
3. 前端消费消息:
```javascript
const socket = new WebSocket("ws://localhost:8080/notification");
socket.onmessage = function(event) {
const message = event.data;
// 处理收到的消息
};
socket.onopen = function() {
const userId = "指定用户的ID";
socket.send(userId);
};
```
4. 后端处理前端消息请求:
```java
@Controller
public class NotificationController {
private final Map<String, WebSocketSession> sessions = new ConcurrentHashMap<>();
@Autowired
private SimpMessagingTemplate messagingTemplate;
@MessageMapping("/notification")
public void notifyUser(String userId, WebSocketSession session) throws IOException {
sessions.put(userId, session);
}
@RabbitListener(queues = "direct_queue")
public void handleMessage(Message<String> message) throws IOException {
String userId = message.getMessageProperties().getReceivedRoutingKey();
String content = message.getBody();
WebSocketSession session = sessions.get(userId);
if (session != null && session.isOpen()) {
messagingTemplate.convertAndSendToUser(session.getId(), "/queue/notification", content);
}
}
}
```
通过以上步骤配置交换机、设置后端生产消息的服务、前端消费消息的WebSocket连接以及后端处理前端消息请求的控制器,可以实现使用Spring Boot和RabbitMQ向指定用户发送通知的功能。请注意替换相关配置和ID,以适应你的实际场景和需求。
### 回答3:
使用Spring Boot和RabbitMQ向指定用户发送通知的实现方式可以分为后端和前端两部分。
后端部分:
1. 首先,在Spring Boot应用配置文件中添加RabbitMQ的连接信息,包括host、port、username、password等。
2. 在后端代码中,通过使用RabbitTemplate向RabbitMQ中的交换机发送消息。首先需要创建一个交换机,使用fanout类型,即广播模式,以确保消息能够被所有的消费者接收到。
```
@Configuration
public class RabbitMQConfig {
@Bean
public FanoutExchange fanoutExchange() {
return new FanoutExchange("message.exchange");
}
@Bean
public RabbitTemplate rabbitTemplate(ConnectionFactory connectionFactory) {
RabbitTemplate rabbitTemplate = new RabbitTemplate(connectionFactory);
rabbitTemplate.setExchange("message.exchange");
return rabbitTemplate;
}
}
@RestController
public class MessageController {
@Autowired
private RabbitTemplate rabbitTemplate;
@GetMapping("/sendMessage/{userId}")
public String sendMessage(@PathVariable String userId, @RequestParam String message) {
rabbitTemplate.convertAndSend(message);
return "发送成功";
}
}
```
3. 在需要发送通知的地方,调用`rabbitTemplate.convertAndSend`发送消息,其中第一个参数为消息内容。
前端部分:
1. 首先,在前端代码中引入RabbitMQ的绑定库,例如STOMP.js。
2. 在前端代码中,使用STOMP.js与RabbitMQ建立WebSocket连接,并订阅接收消息。
```
var socket = new SockJS('/message');
var stompClient = Stomp.over(socket);
stompClient.connect({}, function(frame) {
stompClient.subscribe('/exchange/message.exchange', function(message) {
var content = JSON.parse(message.body).content;
// 处理接收到的消息
});
});
```
3. 在接收到消息的回调函数里,处理接收到的消息。
需要说明的是,这只是一种实现方式,实际项目中需要根据具体需求进行更详细的配置和调整。
相关推荐
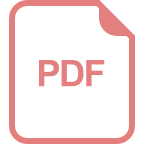
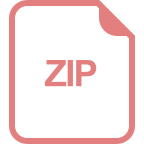











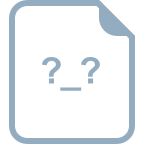
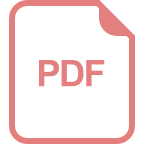