用c sharp 写出蚁群算法
时间: 2023-05-28 10:06:57 浏览: 47
以下是一份使用C#实现的蚁群算法代码,实现了TSP(旅行商问题)的求解:
```
using System;
namespace AntColonyOptimization
{
class Program
{
static void Main(string[] args)
{
int cityCount = 10; // 城市数目
int antCount = 10; // 蚂蚁数目
double alpha = 1; // alpha参数
double beta = 2; // beta参数
double rho = 0.5; // 信息素挥发速度
double Q = 100; // 信息素强度
int maxIter = 100; // 最大迭代次数
// 城市距离矩阵
double[,] distMatrix = new double[cityCount, cityCount]
{
{ 0, 30, 84, 56, 70, 63, 42, 58, 83, 12 },
{ 30, 0, 54, 84, 53, 37, 82, 45, 62, 42 },
{ 84, 54, 0, 43, 96, 77, 12, 69, 38, 93 },
{ 56, 84, 43, 0, 44, 65, 57, 78, 72, 37 },
{ 70, 53, 96, 44, 0, 53, 69, 42, 21, 76 },
{ 63, 37, 77, 65, 53, 0, 57, 54, 88, 24 },
{ 42, 82, 12, 57, 69, 57, 0, 21, 45, 78 },
{ 58, 45, 69, 78, 42, 54, 21, 0, 68, 32 },
{ 83, 62, 38, 72, 21, 88, 45, 68, 0, 56 },
{ 12, 42, 93, 37, 76, 24, 78, 32, 56, 0 }
};
// 初始化信息素矩阵
double[,] pheromoneMatrix = new double[cityCount, cityCount];
for (int i = 0; i < cityCount; i++)
{
for (int j = 0; j < cityCount; j++)
{
pheromoneMatrix[i, j] = 1;
}
}
// 迭代
int iter = 0;
Random random = new Random();
while (iter < maxIter)
{
// 初始化蚂蚁
Ant[] ants = new Ant[antCount];
for (int i = 0; i < antCount; i++)
{
ants[i] = new Ant(cityCount);
}
// 蚂蚁搜索路径
for (int i = 0; i < antCount; i++)
{
for (int j = 0; j < cityCount - 1; j++)
{
int currCity = ants[i].tour[j];
int nextCity = ants[i].nextCity(currCity, pheromoneMatrix, distMatrix, alpha, beta, random);
ants[i].tour[j + 1] = nextCity;
}
}
// 更新信息素
for (int i = 0; i < cityCount; i++)
{
for (int j = 0; j < cityCount; j++)
{
double deltaPheromone = 0;
for (int k = 0; k < antCount; k++)
{
if (ants[k].isVisited(i, j))
{
deltaPheromone += Q / distMatrix[i, j];
}
}
pheromoneMatrix[i, j] = (1 - rho) * pheromoneMatrix[i, j] + deltaPheromone;
}
}
// 寻找最优解
int[] bestTour = ants[0].tour;
double bestDistance = ants[0].tourLength(distMatrix);
for (int i = 1; i < antCount; i++)
{
double distance = ants[i].tourLength(distMatrix);
if (distance < bestDistance)
{
bestTour = ants[i].tour;
bestDistance = distance;
}
}
// 输出当前迭代的最优解
Console.WriteLine("Iter: " + iter + "\tBest Distance: " + bestDistance);
iter++;
}
Console.ReadKey();
}
}
class Ant
{
public int[] tour; // 当前蚂蚁的路径
public bool[] visited; // 当前蚂蚁是否访问过城市
public int visitedCount; // 当前蚂蚁已访问城市数目
public Ant(int cityCount)
{
tour = new int[cityCount];
visited = new bool[cityCount];
visitedCount = 0;
int startCity = new Random().Next(cityCount); // 随机选择一个起始城市
tour[0] = startCity;
visited[startCity] = true;
visitedCount++;
}
// 计算下一个城市
public int nextCity(int currCity, double[,] pheromoneMatrix, double[,] distMatrix, double alpha, double beta, Random random)
{
double[] probabilities = new double[visited.Length];
double sum = 0;
for (int i = 0; i < visited.Length; i++)
{
if (!visited[i])
{
probabilities[i] = Math.Pow(pheromoneMatrix[currCity, i], alpha) * Math.Pow(1.0 / distMatrix[currCity, i], beta);
sum += probabilities[i];
}
}
double r = random.NextDouble() * sum;
double tempSum = 0;
for (int i = 0; i < visited.Length; i++)
{
if (!visited[i])
{
tempSum += probabilities[i];
if (tempSum >= r)
{
visited[i] = true;
visitedCount++;
tour[visitedCount - 1] = i;
return i;
}
}
}
// 如果所有城市都已经访问过,则返回起始城市
for (int i = 0; i < visited.Length; i++)
{
if (!visited[i])
{
visited[i] = true;
visitedCount++;
tour[visitedCount - 1] = i;
return i;
}
}
return -1;
}
// 判断两个城市是否被访问过
public bool isVisited(int city1, int city2)
{
int index1 = Array.IndexOf(tour, city1);
int index2 = Array.IndexOf(tour, city2);
return Math.Abs(index1 - index2) == 1 || Math.Abs(index1 - index2) == tour.Length - 1;
}
// 计算路径长度
public double tourLength(double[,] distMatrix)
{
double length = 0;
for (int i = 0; i < tour.Length - 1; i++)
{
length += distMatrix[tour[i], tour[i + 1]];
}
length += distMatrix[tour[tour.Length - 1], tour[0]];
return length;
}
}
}
```
相关推荐
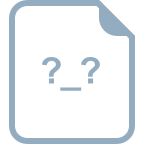
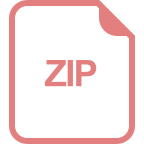
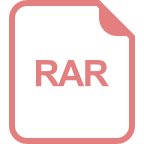

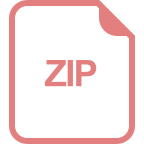
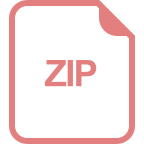
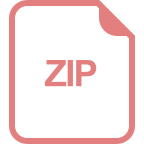
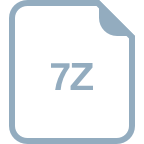
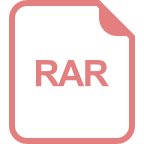
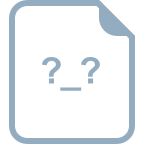
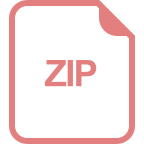
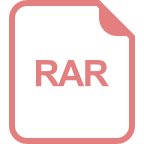
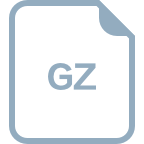
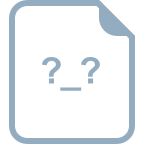