spring boot电商项目分页查询
时间: 2024-08-30 16:01:11 浏览: 62
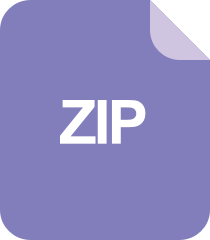
基于Spring Boot的Xmall电商管理系统.zip
Spring Boot 电商项目中的分页查询通常会用到Spring Data JPA或者MyBatis等持久层框架。以下是基本步骤:
1. **引入依赖**:在pom.xml或build.gradle文件中添加Spring Data JPA或MyBatis Pagination插件的依赖。
2. **创建Repository接口**:在Repository接口中声明分页查询方法,比如`Page<T> findAll(Pageable pageable)`,其中`Pageable`是一个用于配置分页信息的对象,包含当前页、每页大小以及排序规则等。
```java
public interface ProductRepository extends JpaRepository<Product, Long> {
Page<Product> findByCategory(Category category, Pageable pageable);
}
```
3. **Service层处理**:在Service中注入对应的Repository,并利用其提供的分页方法获取数据。可以传入用户指定的分页参数,例如页面数、偏移量、排序字段等。
```java
@Service
public class ProductService {
@Autowired
private ProductRepository productRepository;
public Page<Product> getProductListByCategory(Category category, int pageNum, int pageSize) {
Pageable pageable = PageRequest.of(pageNum - 1, pageSize, Sort.by(Sort.Direction.DESC, "createdAt"));
return productRepository.findByCategory(category, pageable);
}
}
```
4. **Controller层展示**:在控制器中接收前端传递的分页参数,调用Service的方法,然后将分页结果返回给前端,前端可以使用jQuery的Datatables、Vue.js的Element UI等库来渲染分页表格。
```java
@RestController
@RequestMapping("/products")
public class ProductController {
@Autowired
private ProductService productService;
@GetMapping("/{category}")
public ResponseEntity<PageResource<Product>> getProductList(@PathVariable("category") String categoryName, @RequestParam(defaultValue="0", value="page") int pageNum, @RequestParam(value="size", defaultValue="10") int pageSize) {
Page<Product> products = productService.getProductListByCategory(Category.findByName(categoryName), pageNum, pageSize);
// 返回分页资源对象
return new ResponseEntity<>(new PageResource<>(products), HttpStatus.OK);
}
}
```
阅读全文
相关推荐
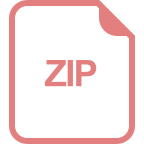
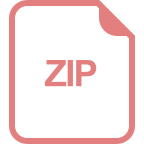
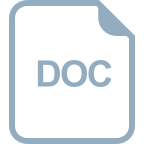
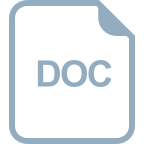
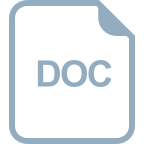
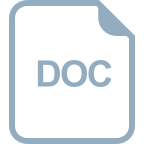
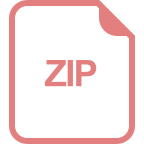
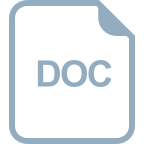
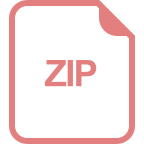
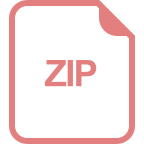
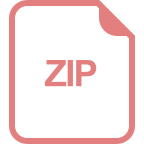
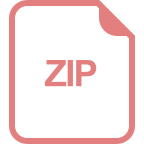
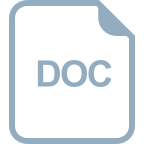
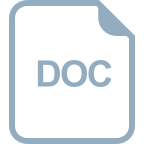
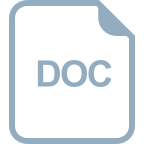
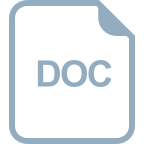
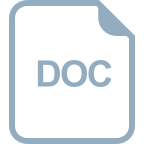
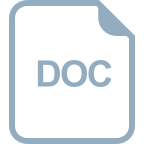