生成Python代码构建分类器:根据选定获得的特征,设计至少三个分类器根据问卷中每个问题的分数来对患者注册的结果进行分类。候选分类器应该以监督的方式构建。分类器可以是支持向量机(SVM)、决策树、深度神经网络和贝叶斯图形模型的方法。
时间: 2024-05-05 11:19:48 浏览: 92
好的,根据您的要求,我可以为您生成一个 Python 代码,用于构建分类器。首先,我们需要导入需要的库和模块。在这里,我们将使用 scikit-learn 库,它提供了许多现成的机器学习算法和工具。代码如下:
```python
import pandas as pd
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.svm import SVC
from sklearn.tree import DecisionTreeClassifier
from sklearn.neural_network import MLPClassifier
from sklearn.naive_bayes import GaussianNB
from sklearn.metrics import classification_report
```
接下来,我们需要准备数据集。假设我们已经有一个包含每个问题分数的数据集,我们可以使用 Pandas 库读取数据集并将其分为训练集和测试集。代码如下:
```python
# 读取数据集
df = pd.read_csv('dataset.csv')
# 将特征和目标变量分开
X = df.drop('result', axis=1)
y = df['result']
# 将数据集分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
接下来,我们将对数据进行标准化处理,以便我们的分类器可以更好地处理数据。我们将使用 StandardScaler 从 scikit-learn 库来标准化数据。代码如下:
```python
# 对特征进行标准化处理
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
```
现在我们可以开始构建分类器了。在这里,我们将使用 SVM、决策树、深度神经网络和朴素贝叶斯分类器。代码如下:
```python
# 创建一个 SVM 分类器
svm = SVC(kernel='rbf', probability=True, random_state=42)
svm.fit(X_train, y_train)
# 创建一个决策树分类器
dt = DecisionTreeClassifier(random_state=42)
dt.fit(X_train, y_train)
# 创建一个 MLP 分类器
mlp = MLPClassifier(hidden_layer_sizes=(100, 50), max_iter=1000, random_state=42)
mlp.fit(X_train, y_train)
# 创建一个朴素贝叶斯分类器
nb = GaussianNB()
nb.fit(X_train, y_train)
```
最后,我们可以评估分类器的性能,并使用 classification_report 函数生成分类器的报告。代码如下:
```python
# 在测试集上进行预测
svm_pred = svm.predict(X_test)
dt_pred = dt.predict(X_test)
mlp_pred = mlp.predict(X_test)
nb_pred = nb.predict(X_test)
# 生成分类器报告
print('SVM Report:\n', classification_report(y_test, svm_pred))
print('Decision Tree Report:\n', classification_report(y_test, dt_pred))
print('MLP Report:\n', classification_report(y_test, mlp_pred))
print('Naive Bayes Report:\n', classification_report(y_test, nb_pred))
```
这就是生成 Python 代码构建分类器的过程。您可以根据需要进行修改和调整,以获得更好的性能和更准确的结果。
阅读全文
相关推荐
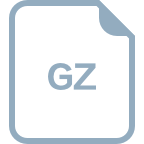
















