shell脚本编写汉诺塔
时间: 2023-11-15 16:02:11 浏览: 68
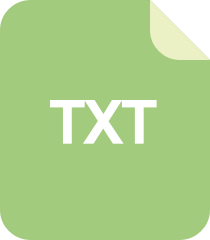
汉诺塔程序代码
汉诺塔是一种经典的递归问题,可以用shell脚本来实现。下面是一个汉诺塔的shell脚本实现:
#! /bin/bash
function hanoi() {
local num=$1
if [ "$num" -eq "1" ];then
echo "Move:$2----->$4"
else
hanoi $((num-1)) $2 $4 $3
echo "Move:$2----->$4"
hanoi $((num-1)) $3 $2 $4
fi
}
hanoi $1 $2 $3 $4
这个脚本中,hanoi函数接受四个参数,分别是盘子的数量、起始柱子、中间柱子和目标柱子。如果盘子数量为1,则直接将盘子从起始柱子移动到目标柱子;否则,先将前n-1个盘子从起始柱子移动到中间柱子,再将最后一个盘子从起始柱子移动到目标柱子,最后将前n-1个盘子从中间柱子移动到目标柱子。
阅读全文
相关推荐
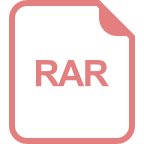
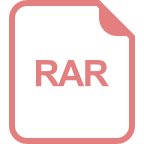
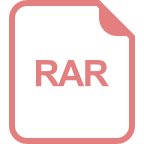
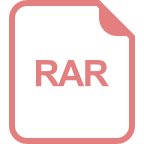
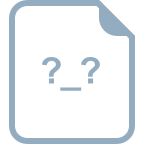









